timePicker
timePicker
lets users enter or select a time.
-
XML element:
timePicker
-
Java class:
TypedTimePicker
Basics
Time can be entered directly using a keyboard or selected from an overlay with a list of time values. The overlay appears on clicking the field or the clock button.
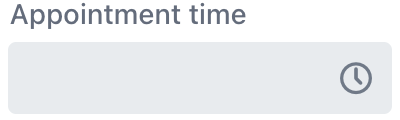
The following example defines a timePicker
of localTime
type with a label:
<timePicker id="timePicker" datatype="localTime" label="Appointment time"/>
Data Types
timePicker
is a typed component which supports common data types for storing a time value:
-
localTime
-
offsetTime
-
time
To change the type, use the datatype attribute.
Step
The default interval between the items displayed in the time overlay is set to one hour. To customize this interval, use the step attribute either in XML or programmatically.
Setting the step
attribute in the view descriptor XML:
<timePicker step="30"
min="10:00"
max="12:00"/> (1)
1 | A number without a suffix means minutes. |
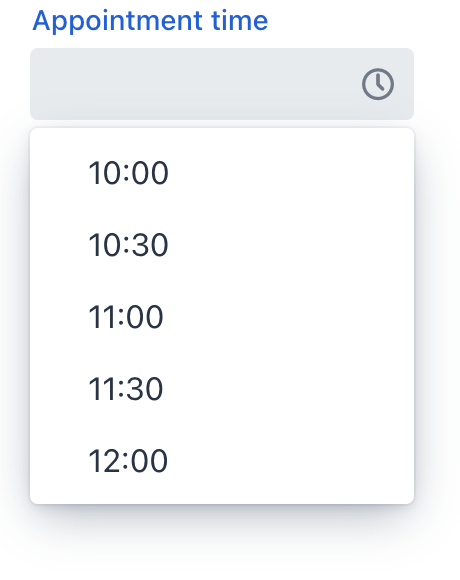
The step
attribute supports flexible time interval configuration using different chrono units. You can specify values with unit suffixes for clarity.
<timePicker step="2h"/> (1)
<timePicker step="1600s"/> (2)
<timePicker step="20m"/> (3)
1 | 2 hour intervals. |
2 | 1600 second (26m 40s) intervals. |
3 | 20 minute intervals. |
This can also be done in the view controller:
@ViewComponent
private TypedTimePicker<Comparable> timePicker;
@Subscribe
public void onInit(InitEvent event) {
timePicker.setStep(Duration.ofMinutes(30));
}
The step must divide an hour or day evenly. For example, "15 minutes", "30 minutes" and "2 hours" are valid steps, whereas "42 minutes" isn’t.
The overlay doesn’t appear for steps less than 15 minutes, to avoid showing an impractical number of choices. |
Theme Variants
Use the themeNames attribute to adjust text alignment, helper text placement, and component size.
Alignment
Choose among three alignment options: align-left
(default), align-right
, align-center
.

XML code
<timePicker themeNames="align-left"/>
<timePicker themeNames="align-center"/>
<timePicker themeNames="align-right"/>
Helper Text Position
Setting helper-above-field
will move the helper text from its default position below the field to above it.
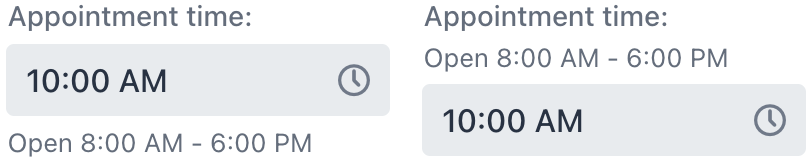
XML code
<timePicker label="Appointment time:" helperText="Open 8:00 AM - 6:00 PM"/>
<timePicker themeNames="helper-above-field" label="Appointment time:" helperText="Open 8:00 AM - 6:00 PM"/>
Attributes
id - alignSelf - allowedCharPattern - ariaLabel - ariaLabelledBy - autoOpen - classNames - clearButtonVisible - colspan - css - dataContainer - datatype - enabled - errorMessage - focusShortcut - height - helperText - label - max - maxHeight - maxWidth - min - minHeight - minWidth - overlayClass - placeholder - property - readOnly - required - requiredMessage - step - tabIndex - themeNames - visible - width
Handlers
AttachEvent - BlurEvent - ClientValidatedEvent - ComponentValueChangeEvent - DetachEvent - FocusEvent - InvalidChangeEvent - TypedValueChangeEvent - statusChangeHandler - validator
To generate a handler stub in Jmix Studio, use the Handlers tab of the Jmix UI inspector panel or the Generate Handler action available in the top panel of the view class and through the Code → Generate menu (Alt+Insert / Cmd+N). |
ClientValidatedEvent
ClientValidatedEvent
is sent by the web component whenever it is validated on the client-side.
InvalidChangeEvent
com.vaadin.flow.component.timepicker.TimePicker.InvalidChangeEvent
is sent when the value of the invalid attribute of the component changes.
validator
Adds a validator instance to the component. The validator must throw ValidationException
if the value is not valid.
@Install(to = "timePicker", subject = "validator")
private void timePickerValidator(LocalTime value) {
if (value != null && LocalTime.of(13,0).isBefore(value) && LocalTime.of(14,0).isAfter(value)) {
throw new ValidationException("No appointments between 13:00 to 14:00.");
}
}
See Also
See Vaadin Docs for more information.