Custom Icons
Additional icons can be added as an SVG sprite or standalone icons and used alongside the built-in sets.
Icon Set
An SVG sprite is a collection of SVG images loaded into an application as a single file, providing performance benefits. You can create a sprite manually or using one of many third-party tools.
Follow the steps below to add an icon set:
-
Place your SVG sprite in the
/frontend/icons/
directory. Themy-icons.js
is used as an example and contains three icons:my-icons.jsimport '@vaadin/icon/vaadin-iconset.js'; const template = document.createElement('template'); template.innerHTML = `<vaadin-iconset name="my-icons" size="24"> <svg><defs> <g id="star"><path d="M12 .587l3.668 7.568 8.332 1.207-6 5.848 1.416 8.263L12 18.896l-7.416 3.895L6 14.162l-6-5.848 8.332-1.207z" fill="#FFD700"/></g> <g id="heart"><path d="M12 21.35l-1.45-1.32C5.4 15.36 2 12.28 2 8.5 2 5.42 4.42 3 7.5 3c1.74 0 3.41.81 4.5 2.09C13.09 3.81 14.76 3 16.5 3 19.58 3 22 5.42 22 8.5c0 3.78-3.4 6.86-8.55 11.54L12 21.35z" fill="#FF69B4"/></g> <g id="circle"><circle cx="12" cy="12" r="10" fill="#1E90FF"/></g> </defs></svg> </vaadin-iconset>`; document.head.appendChild(template.content);
-
Create an enum that implements
IconFactory
:MyIcons.javapackage com.company.onboarding.icons; import com.vaadin.flow.component.dependency.JsModule; import com.vaadin.flow.component.icon.IconFactory; import java.util.Locale; @JsModule("./icons/my-icons.js") public enum MyIcons implements IconFactory { STAR, HEART, CIRCLE; public Icon create() { return new Icon(this.name().toLowerCase(Locale.ENGLISH).replace('_', '-').replaceAll("^-", "")); } public static final class Icon extends com.vaadin.flow.component.icon.Icon { Icon(String icon) { super("my-icons", icon); } } }
Custom icons can now be used in the same way as the default ones:
<icon icon="my-icons:star"/>
<icon icon="my-icons:heart"/>
<icon icon="my-icons:circle"/>
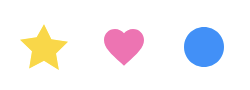
Or set it to a component in the Java code:
spriteIconButton.setIcon(MyIcons.STAR.create());
Standalone Icon
Standalone icons can be added to the static content directory. By default, Spring Boot serves static resources from the following locations in the classpath: /static
, /public
, /resources
, or /META-INF/resources
. To help organize icons, you may set a subfolder META-INF/resources/icons
.
To serve a static resource, use the svgIcon
component:
<svgIcon resource="/icons/tree.svg"/>
To set the icon to a component, use its prefix or suffix elements. For example, to put the icon before the button text:
<button text="Click!">
<prefix>
<svgIcon resource="/icons/tree.svg"/>
</prefix>
</button>
Or, use the programmatic approach:
StreamResource iconResource = new StreamResource("tree.svg",
() -> getClass().getResourceAsStream("/META-INF/resources/icons/tree.svg"));
SvgIcon treeIcon = new SvgIcon(iconResource);
standaloneIconButton.setIcon(treeIcon);