html
Jmix includes a set of HTML components for standard HTML elements. If no suitable component is included, or you want to include native HTML directly, use the html
component. It can render HTML provided as a string, CDATA, or file.
-
XML element:
html
-
Java class:
Html
Basics
This element is designed for embedding HTML within an XML structure. It allows embedding separate HTML elements or blocks of HTML code. The block must consist of a single root element such as <header>
, <footer>
, <main>
, or <div>
. Within this root element can be any number of nested <div>
elements to structure the content effectively.
Note that it is the developer’s responsibility to sanitize and remove any dangerous parts of the HTML before sending it to the user through this component. Passing raw input data to the user can possibly lead to cross-site scripting attacks. |
Message Bundle
This example demonstrates how to add HTML content via a string in the message bundle:
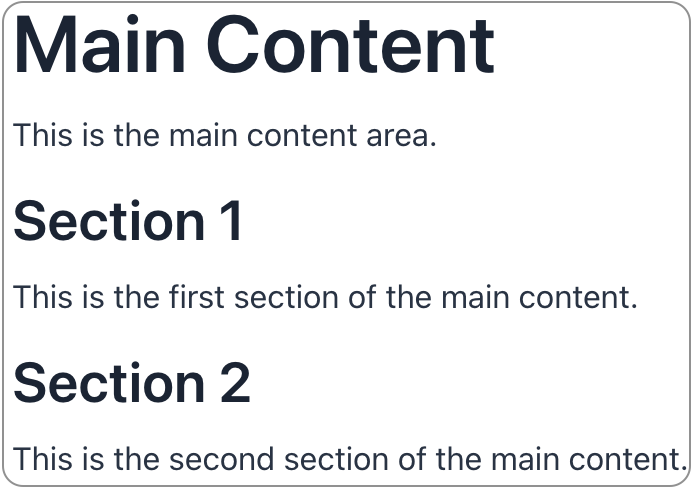
<html content="msg://html.content"/>
com.company.onboarding.view.component.html/html.content=\
<main>\
<h1>Main Content</h1>\
<p>This is the main content area.</p>\
<div>\
<h2>Section 1</h2>\
<p>This is the first section of the main content.</p> \
</div>\
<div>\
<h2>Section 2</h2>\
<p>This is the second section of the main content.</p>\
</div>\
</main>
Character Data
The same HTML block can be added within a CDATA (Character Data) section:
<html id="html">
<content>
<![CDATA[
<main>
<h1>Main Content</h1>
<p>This is the main content area.</p>
<div>
<h2>Section 1</h2>
<p>This is the first section of the main content.</p>
</div>
<div>
<h2>Section 2</h2>
<p>This is the second section of the main content.</p>
</div>
</main>
]]>
</content>
</html>
File Content
The code for HTML block can be also obtained from a file using one of the following methods.
Static Resource
The file can be served statically by the application. If your file is located
at /src/main/resources/META-INF/resources/html/html-file.html
, specify it as follows:
<html id="staticHtml" file="META-INF/resources/html/html-file.html"/>
By default, static content is served from /static , /public , /resources , or /META-INF/resources directories of the classpath. See details in the Spring Boot documentation.
|
Stream Resource
The HTML code can be supplied as a file through the InputStream
, and then the component can be initialized by passing it in.
The component cannot be created using the UiComponents bean, as it does not provide a no-arguments constructor.
|
protected static final String SRC_PATH = "META-INF/resources/html/html-file.html";
@Autowired
private Resources resources;
@Subscribe
public void onInit(final InitEvent event) {
InputStream resourceAsStream = resources.getResourceAsStream(SRC_PATH);
Html html = new Html(resourceAsStream);
}
CSS Properties
Use the css attribute to add CSS properties declaratively:
<html file="META-INF/resources/html/html-file.html" css="background-color: grey;"/>
Alternatively, you can add custom CSS styling within the application’s theme.
Handlers
To generate a handler stub in Jmix Studio, use the Handlers tab of the Jmix UI inspector panel or the Generate Handler action available in the top panel of the view class and through the Code → Generate menu (Alt+Insert / Cmd+N). |