Service Task
Flowable engine gives you the following ways to declare how Java logic should be invoked for the service task:
-
Specifying a class that implements
JavaDelegate
orActivityBehavior
. -
Evaluating an expression that resolves to a delegation object.
-
Invoking a method expression.
See Flowable documentation for details.
Spring Bean Service Task
BPM add-on adds one more way to define a service task. It allows you to select a Spring bean, the bean method and provide parameter values for the selected method. Bean name and methods are selected from drop-down lists. After the method is selected, a panel for entering method argument values is displayed.
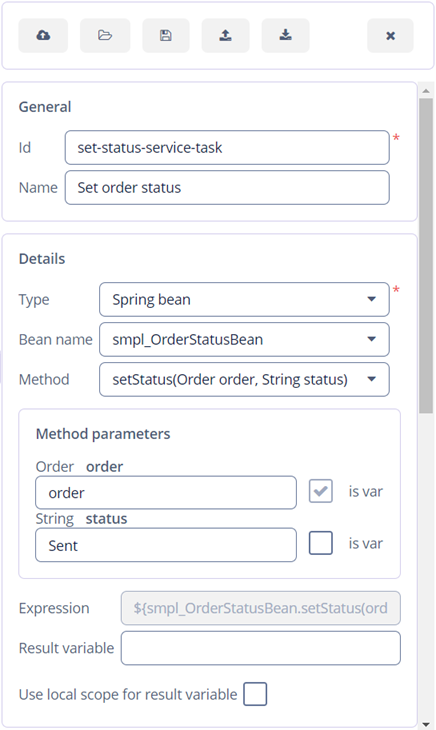
The panel for the Spring bean helps to build an expression for bean method invocation. In case of method from the screenshot above, the expression will be:
${smpl_OrderStatusBean.setStatus(order, 'Sent')}
Pay attention to the is var check box. It makes sense mostly for string parameters. If the check box is not selected, then the argument value will be written to the resulting expression in apostrophes. If the check box is selected, no apostrophes will be added and a variable with a provided name will be passed to the method.
-
${smpl_MyBean.someMethod('description')}
– this expression will use the string valuedescription
. -
${smpl_MyBean.someMethod(description)}
– this expression will use the value of the variable nameddescription
.
Java Delegate Service Task
If you select JavaDelegate class
in the Type combo box, then the list of classes that implement the org.flowable.engine.delegate.JavaDelegate
interface will appear. See Flowable documentation for more details.
If the selected JavaDelegate class contains fields of org.flowable.common.engine.api.delegate.Expression
type (see Field Injection in Flowable documentation), then field names will appear in the Fields table.
In case you want to use Spring context in the JavaDelegate
implementation, add @Component
annotation and select Delegate expression
in the modeler.
If you use Flowable field injection in JavaDelegate
with Spring context, then this bean scope must be prototype
- you need to add the @Scope(BeanDefinition.SCOPE_PROTOTYPE)
annotation. See Flowable documentation for details.
Here is an example of Java class that sends an email:
@Component("smpl_SendEmailJavaDelegate")
@Scope(BeanDefinition.SCOPE_PROTOTYPE)
public class SendEmailJavaDelegate implements JavaDelegate {
private Expression addressee; (1)
private Expression emailSubject;
private Expression emailBody;
@Autowired
private Emailer emailer;
@Override
public void execute(DelegateExecution execution) { (2)
User addresseeValue = (User) addressee.getValue(execution); (3)
String emailSubjectValue = (String) emailSubject.getValue(execution);
String emailBodyValue = (String) emailBody.getValue(execution);
EmailInfo emailInfo = EmailInfoBuilder.create() (4)
.setAddresses(addresseeValue.getEmail())
.setSubject(emailSubjectValue)
.setFrom(null)
.setBody(emailBodyValue)
.build();
emailer.sendEmailAsync(emailInfo); (5)
}
}
1 | We declare three fields. Values of the fields are defined in the process model. |
2 | The execute method is invoked when the process reaches the service task. |
3 | Evaluate the value of the expression. |
4 | Creates EmailInfo object. |
5 | Sends email asynchronously. |
The properties panel for the service task looks this way:
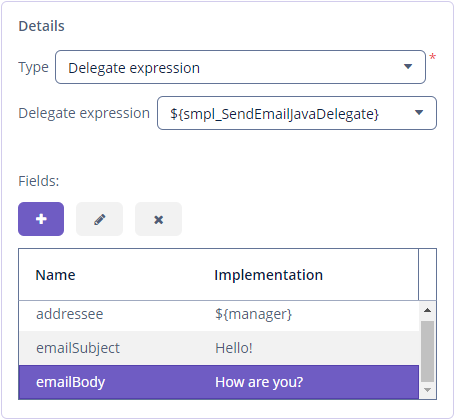
You can specify fields in the editor:
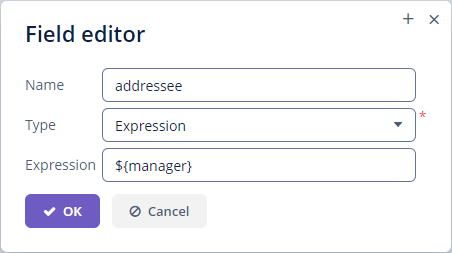
In the example above, we select the expression
field type for the addressee
field. In our case, the manager
process variable contains a user.