Views
A view is a basic building block of the application UI. It is a root of the hierarchy of visual components and can also contain data components, actions and facets.
A view is defined by a Java class. The class usually has a corresponding XML file called descriptor, which defines the view content. So there is a clear separation between declarative layout of components defined in XML and programmatic initialization and event handling logic written in Java.
View Classes
Jmix provides the following base classes for views:
StandardMainView
StandardMainView
is a root application view which is opened after login. It contains the AppLayout component with the main menu.
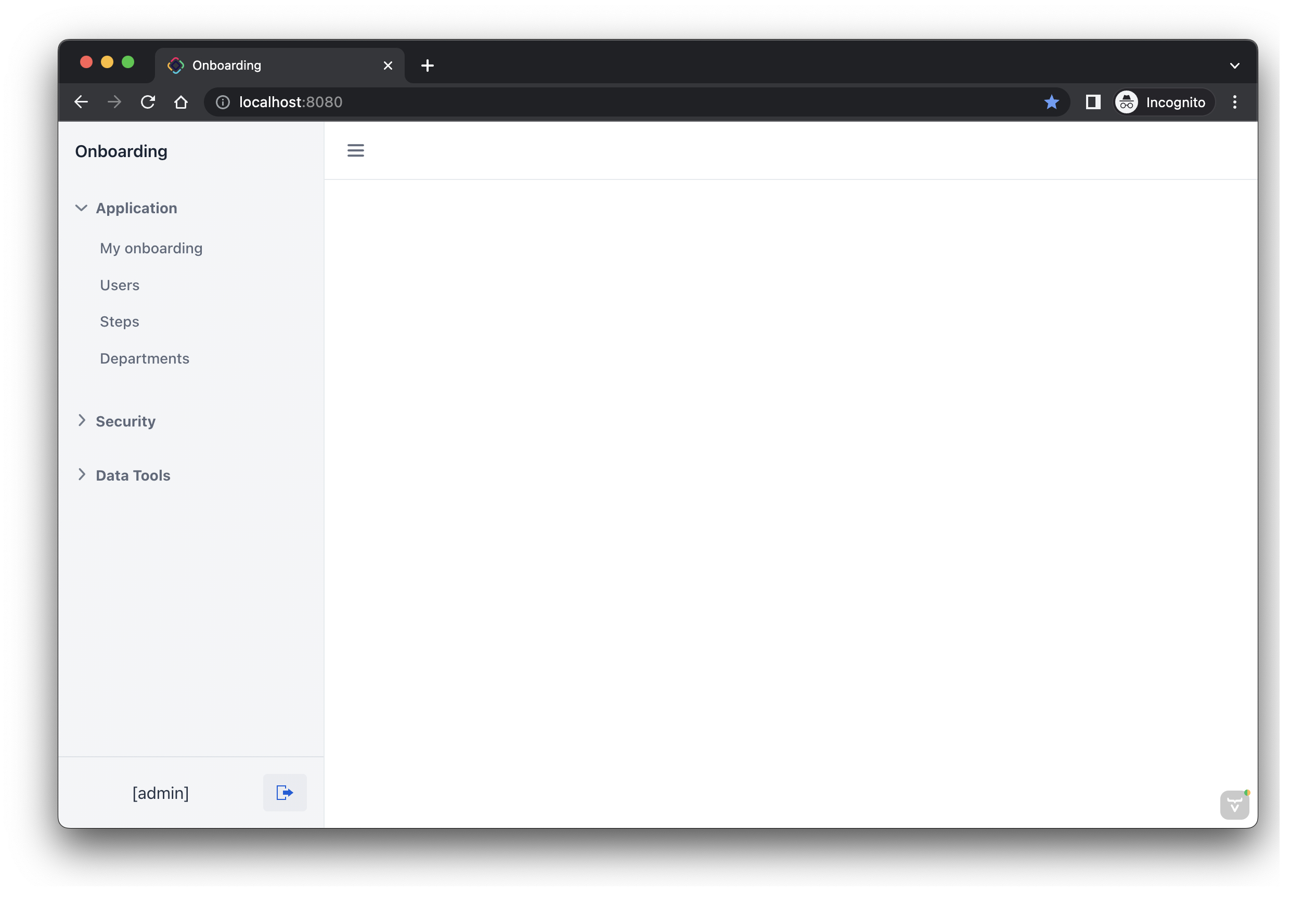
StandardView
StandardView
is a base class for regular views. Such views can be shown either inside the main view using navigation to the view URL or in a popup dialog window.
Below is a standard view opened using the /my-onboarding
URL:
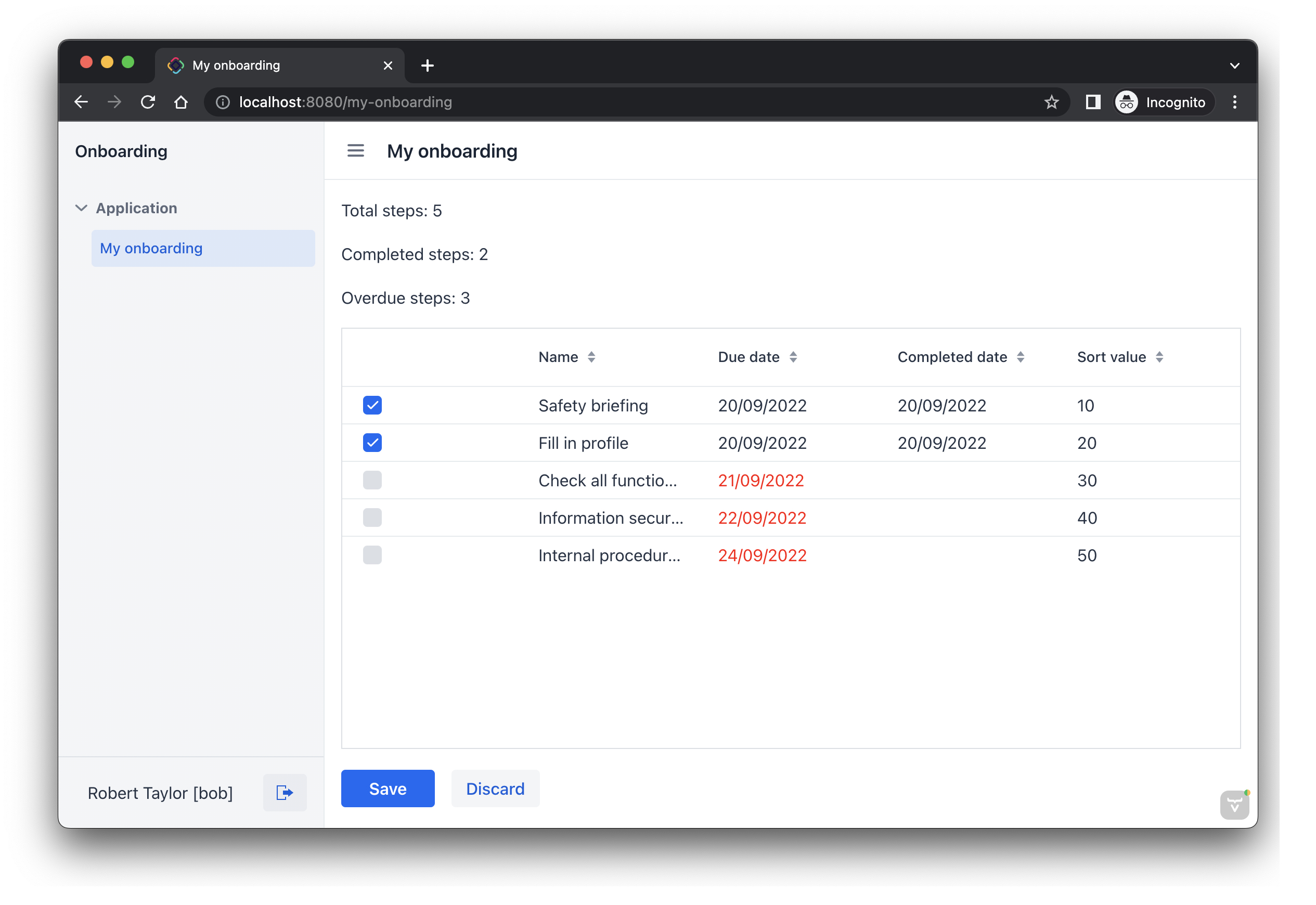
StandardListView
StandardListView
is a base class for CRUD views displaying a list of entities. It can also be used as a lookup view to select entity instances from the list and return them to the caller. A list view usually uses the dataGrid or treeDataGrid components.
Below is a list view opened using the /departments
URL:
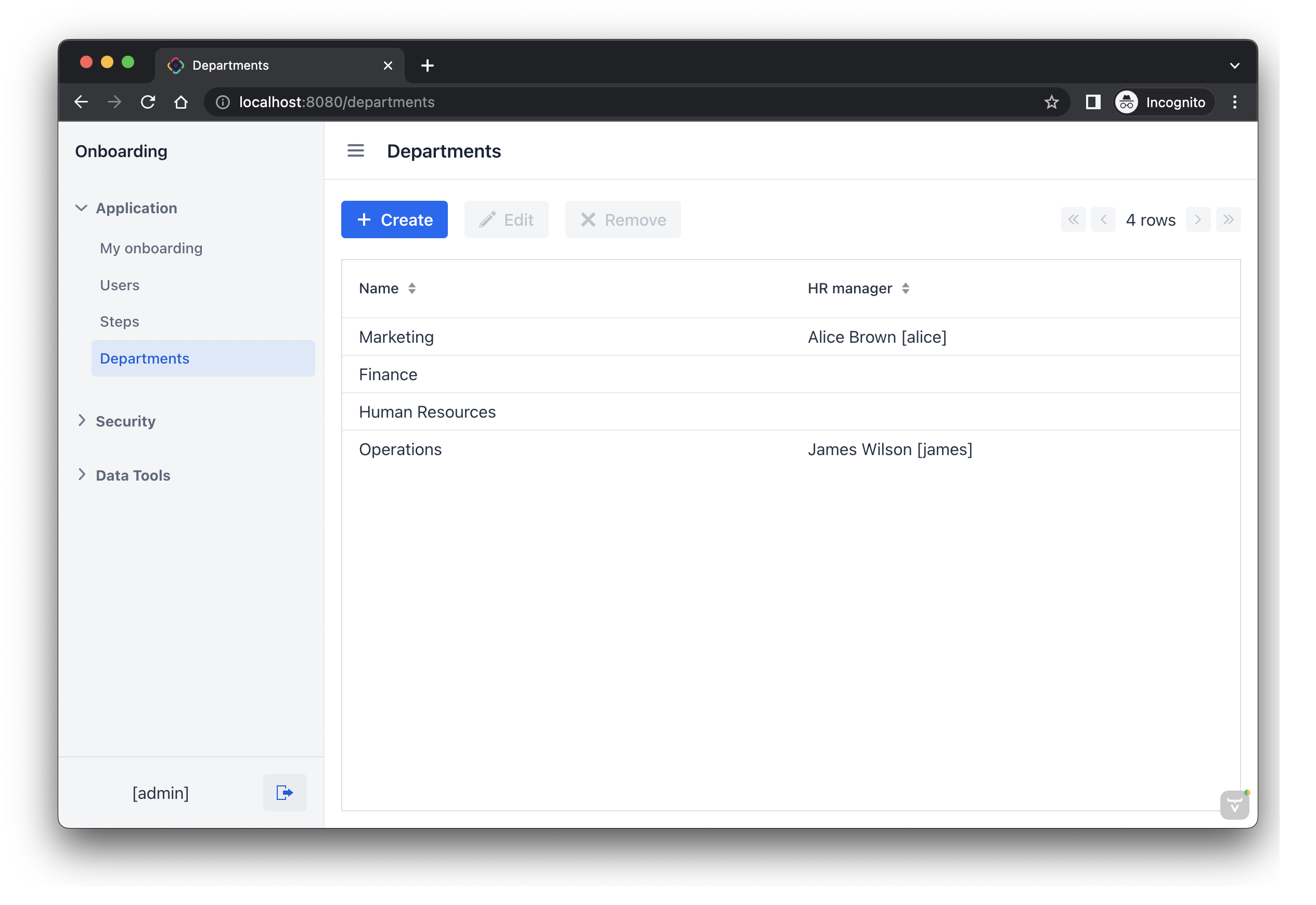
The same view opened in a dialog for selecting entities:
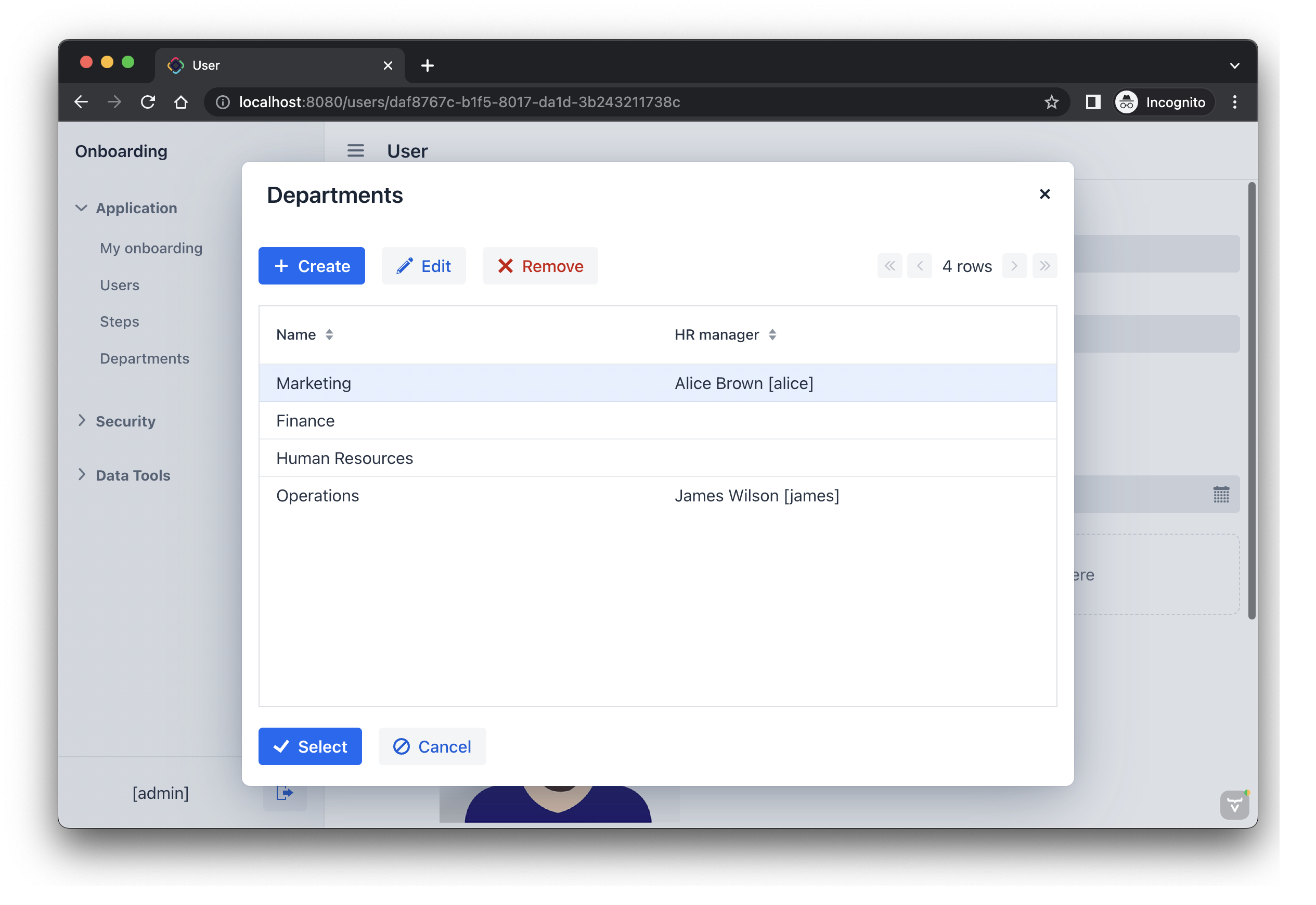
StandardDetailView
StandardDetailView
is a base class for CRUD views displaying a single entity instance. A detail view usually uses the formLayout component.
Below is a detail view opened using entity id in the URL:
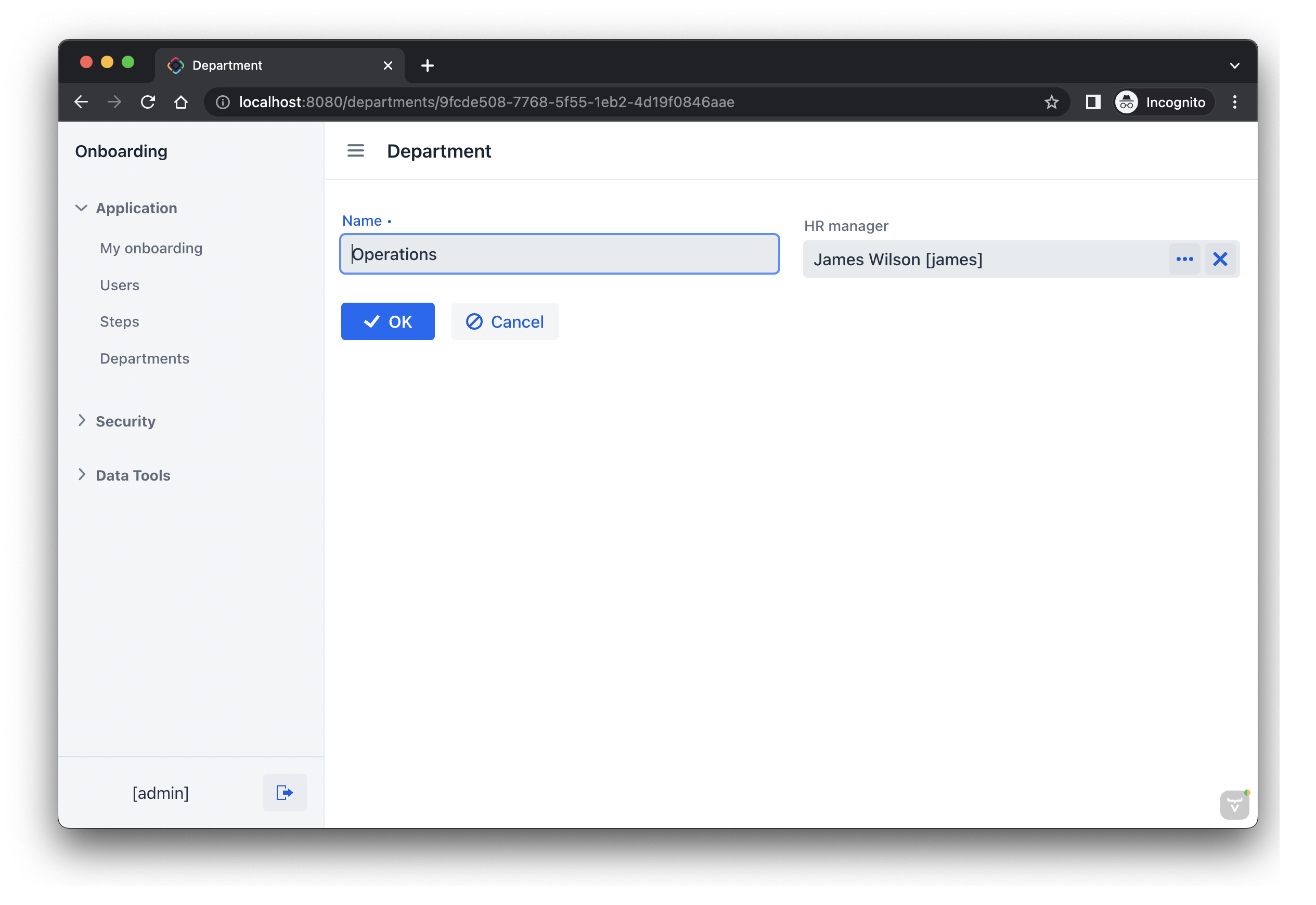
The same view opened in a dialog:
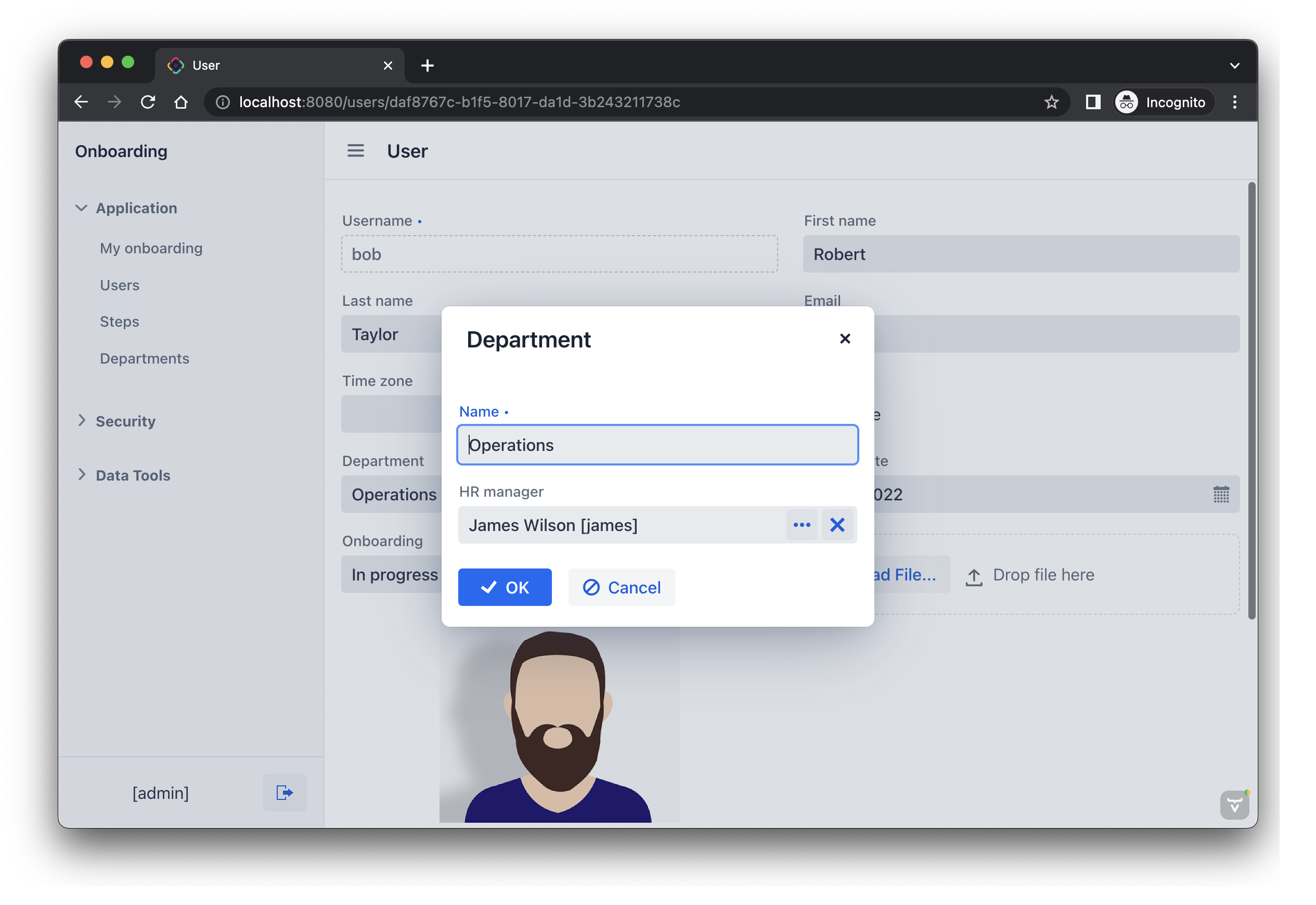
View Annotations
Annotations on view classes provide information about the views to the framework. Some annotations are applicable to any type of view, some of them can be used only on entity list or detail views.
Common Annotations
@ViewController("MyOnboardingView")
@ViewDescriptor("my-onboarding-view.xml")
@Route(value = "my-onboarding", layout = MainView.class)
@DialogMode(width = "AUTO", height = "AUTO")
public class MyOnboardingView extends StandardView {
-
@ViewController
annotation indicates that the class is a view. The value of the annotation is the view id which can be used to refer to the view from the main menu or when opening the view programmatically. -
@ViewDescriptor
annotation connects the view class to the XML descriptor. The value of the annotation specifies the path to the descriptor file. If the value contains a file name only, it is assumed that the file is located in the same package as the view class. -
@com.vaadin.flow.router.Route
defines a URL path for navigation to this view. As long as the view should be opened inside the main view, thelayout
attribute specifies the main view class. -
@DialogMode
annotation defines parameters of the dialog window when the view is opened as a dialog. -
@com.vaadin.flow.server.auth.AnonymousAllowed
makes the view available without authentication. By default, only the login view is available to the anonymous (not authenticated) session.See the User Registration sample project for how the @AnonymousAllowed
annotation is used in a view for self-registration of users.
List View Annotations
// common annotations
@ViewController("Department.list")
@ViewDescriptor("department-list-view.xml")
@Route(value = "departments", layout = MainView.class)
@DialogMode(width = "50em", height = "37.5em")
// list view annotations
@LookupComponent("departmentsTable")
@PrimaryLookupView(Department.class)
public class DepartmentListView extends StandardListView<Department> {
-
@LookupComponent
annotation specifies an id of a UI component to be used for getting a value returned from the lookup. -
@PrimaryLookupView
annotation indicates that this view is the default lookup view for the specified entity. The annotation has greater priority than the{entity_name}.lookup
/{entity_name}.list
name convention.
Detail View Annotations
// common annotations
@ViewController("Department.detail")
@ViewDescriptor("department-detail-view.xml")
@Route(value = "departments/:id", layout = MainView.class)
// detail view annotations
@EditedEntityContainer("departmentDc")
@PrimaryDetailView(Department.class)
public class DepartmentDetailView extends StandardDetailView<Department> {
-
@EditedEntityContainer
annotation specifies a data container that contains the edited entity. -
@PrimaryDetailView
annotation indicates that this view is the default detail view for the specified entity. The annotation has greater priority than the{entity_name}.detail
name convention.