ProgressBar
ProgressBar
shows the completion status of a process. The progress can be determinate or indeterminate. Use ProgressBar
to show an ongoing process that takes a noticeable time to finish.

Component’s XML-name: progressBar
.
Determinate Process
For the example below, we will use the background tasks mechanism.
Let’s simulate a situation where you can track the current stage of the process.
<progressBar id="progressBar" width="100%" />
@Autowired
protected ProgressBar progressBar;
@Autowired
protected BackgroundWorker backgroundWorker;
private static final int ITERATIONS = 6;
@Subscribe
protected void onInit(InitEvent event) {
BackgroundTask<Integer, Void> task = new BackgroundTask<Integer, Void>(100) {
@Override
public Void run(TaskLifeCycle<Integer> taskLifeCycle) throws Exception {
for (int i = 1; i <= ITERATIONS; i++) {
TimeUnit.SECONDS.sleep(1); (1)
taskLifeCycle.publish(i);
}
return null;
}
@Override
public void progress(List<Integer> changes) {
double lastValue = changes.get(changes.size() - 1);
progressBar.setValue(lastValue / ITERATIONS); (2)
}
};
BackgroundTaskHandler taskHandler = backgroundWorker.handle(task);
taskHandler.execute();
}
1 | Some task that takes time to complete |
2 | Setting the value of the component, it should be a double number between 0.0 and 1.0 |
Indeterminate Process
If a running process cannot send information about the progress, the ProgressBar
can display an indeterminate state of the indicator. To show an indeterminate state, set the indeterminate
attribute to true
. The default value is false
.
For example:
<progressBar indeterminate="true" width="200px"/>
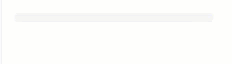
Styling
To make the progress bar indicator appear as a dot that progresses over the progress bar track instead of a growing bar, uses stylename
attribute with point
value.

By default, an indeterminate progress bar is displayed as a horizontal bar. To make it a spinning wheel, uses stylename
attribute with indeterminate-circle
value.
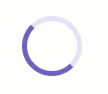
Events and Handlers
To generate a handler stub in Jmix Studio, select the component in the screen descriptor XML or in the Jmix UI hierarchy panel and use the Handlers tab of the Jmix UI inspector panel. Alternatively, you can use the Generate Handler button in the top panel of the screen controller. |
ValueChangeEvent
ValueChangeEvent<Double>
is sent on the value changes and has the following methods:
-
getValue() - returns number between
0.0
and1.0
that represents the current progress state, where1.0
is the process completed and0.0
is not started. -
getPrevValue() - returns the same values but represents the state of the process at the previous stage.
Programmatic registration of the event handler: use the addValueChangeListener()
component method.
All XML Attributes
You can view and edit attributes applicable to the component using the Jmix UI inspector panel of the Studio’s Screen Designer. |
align - box.expandRatio - caption - captionAsHtml - colspan - contextHelpText - contextHelpTextHtmlEnabled - css - description - descriptionAsHtml - enable - height - htmlSanitizerEnabled - icon - id - indeterminate - responsive - rowspan - stylename - visible - width