URL Routes Generator
Sometimes, it is necessary to get a proper URL of some application screen that can be sent via email or shown to the user. The simplest way to generate it is by using the URL Routes Generator.
URL Routes Generator provides API for generating links to an entity editor screen or a screen defined by its id or class. The link can also contain URL parameters that enable to reflect inner screen state to URL to use it later.
The getRouteGenerator()
method of UrlRouting
bean allows you to get an instance of RouteGenerator
. RouteGenerator
has the following methods:
-
getRoute(String screenId)
- returns a route for a screen with the givenscreenId
, for example:String route = urlRouting.getRouteGenerator().getRoute("uiex1_Customer.browse");
The resulting URL will be like
http://host:port/#main/customers
-
getRoute(Class<? extends Screen> screenClass)
- generates a route for a screen with the givenscreenClass
, for example:String route = urlRouting.getRouteGenerator().getRoute(CustomerBrowse.class);
The resulting URL will be like
http://host:port/#main/customers
-
getEditorRoute(Object entity)
- generates a route to a default editor screen of the given entity, for example:Customer e = customerField.getValue(); String route = urlRouting.getRouteGenerator().getEditorRoute(e);
The resulting URL will be like
http://host:port/#main/customers/edit?id=5jqtc3pwzx6g6mq1vv5gkyjn0s
-
getEditorRoute(Object entity, Class<? extends Screen> screenClass)
- generates a route for an editor with the givenscreenClass
andentity
. -
getRoute(Class<? extends Screen> screenClass, Map<String, String> urlParams)
- generates a route for a screen with the givenscreenClass
andurlParams
.
URL Routes Generator Example
Suppose we have a Customer
entity with standard screens that have registered routes. Let’s add a button to the browser screen that generates a link to the editor of the selected entity:
@Autowired
protected UrlRouting urlRouting;
@Autowired
protected Dialogs dialogs;
@Subscribe("getLinkButton")
protected void onGetLinkButtonClick(Button.ClickEvent event) {
Customer selectedCustomer = customersTable.getSingleSelected();
if (selectedCustomer != null) {
String routeToSelectedRole = urlRouting.getRouteGenerator()
.getEditorRoute(selectedCustomer);
dialogs.createMessageDialog()
.withCaption("Generated route")
.withMessage(routeToSelectedRole)
.withWidth("710")
.show();
}
}
The resulting route looks like this:
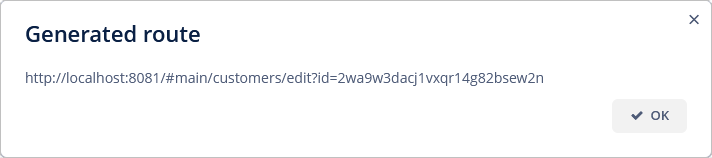