Form
Form
component is designed for the joint display and editing of multiple entity attributes.
Component’s XML-name: form
.
Basics
Form
is a simple container similar to GridLayout
. You can define any number of nested columns and fields declaratively in XML. Field captions are located to the left of the fields. The main difference from GridLayout
is that Form
enables binding all nested fields to one data container.
Usage example:
<data>
<instance id="orderDc"
class="ui.ex1.entity.Order"
fetchPlan ="_base"/>
</data>
<layout>
<form dataContainer="orderDc">
<dateField property="date"/>
<textField property="amount"
description="Total amount"/>
<entityPicker property="customer"/>
<field id="ratingField" property="rating"/>
</form>
</layout>
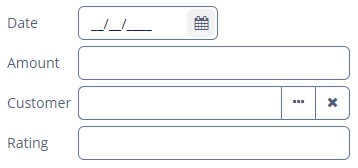
In the example above, the Form
component shows attributes of the entity loaded into the orderDc
data container. Nested form
elements define visual components bound to entity attributes using the property
XML attribute. Captions will be created automatically based on the localized names of entity attributes.
Apart from concrete visual components, the Form
can also contain generic fields defined with the nested field
element. The framework will choose an appropriate visual component based on the corresponding entity attribute and existing component generation strategies.
The nested components can have any common or specific attributes like the description
shown in the example. To add a nested component to the screen controller, give it the id
attribute in XML.
To add |
Attributes
-
dataContainer
- sets the data container for nested fields. -
captionPosition
- defines the fields caption position:TOP
orLEFT
. -
childrenCaptionAlignment
– defines the alignment of child component captions in all nested columns. Two options are available:LEFT
andRIGHT
. The default value isLEFT
. Applicable only when thecaptionPosition
attribute isLEFT
. -
childrenCaptionWidth
– specifies fixed captions width for all nested columns and their child elements. Set-1
to use auto size. -
colspan
androwspan
:The
Form
component supports thecolspan
androwspan
attributes. These attributes set the number of columns and rows occupied by the corresponding nested component. An example below demonstrates howField 1
can be extended to cover two columns:<layout> <form> <column width="250px"> <textField caption="Field 1" colspan="2" width="100%"/> <textField caption="Field 2"/> </column> <column width="250px"> <textField caption="Field 3"/> </column> </form> </layout>
Similarly,
Field 1
can be extended to cover two rows:<layout> <form> <column width="250px"> <textField caption="Field 1" rowspan="2" height="100%"/> </column> <column width="250px"> <textField caption="Field 2"/> <textField caption="Field 3"/> </column> </form> </layout>
Methods
-
setChildrenCaptionAlignment(CaptionAlignment alignment)
– sets alignment of child component captions in all columns. -
setChildrenCaptionAlignment(int column, CaptionAlignment alignment)
– sets alignment of child component captions for a column with the given index. -
add()
- enables adding fields to theForm
programmatically:It takes a
Component
instance as a parameter, and you can also define the position of the new field by addingcolumn
androw
indexes. Besides, an overloaded method can takerowspan
andcolspan
parameters.Data container is not assigned to the components added programmatically, so you have to use the component’s
setValueSource()
method for data binding.For example:
<data> <instance id="customerDc" class="ui.ex1.entity.Customer" fetchPlan ="_base"/> </data> <layout> <form id="form" dataContainer="customerDc"> <column> <textField id="nameField" property="firstName"/> </column> </form> </layout>
You can add an
email
field to the form programmatically in the screen controller as follows:@Autowired private UiComponents uiComponents; @Autowired private InstanceContainer<Customer> customerDc; @Autowired private Form form; @Subscribe private void onInit(InitEvent event) { TextField<String> emailField = uiComponents.create(TextField.TYPE_STRING); emailField.setCaption("Email"); emailField.setWidthFull(); emailField.setValueSource(new ContainerValueSource<>(customerDc, "email")); form.add(emailField); }
Column Element
Column
is an optional element that allows you to position fields in multiple columns. For this purpose, nested fields should be placed not immediately within the form
, but within a column
. For example:
<layout>
<form dataContainer="orderDc">
<column width="250px">
<dateField property="date"/>
<textField property="amount"/>
</column>
<column width="400px">
<entityPicker property="customer"/>
<textArea property="num"/>
</column>
</form>
</layout>
In this case, fields will be arranged in two columns; the first column will contain all fields with a width of 250px
, the second one with a width of 400px
.
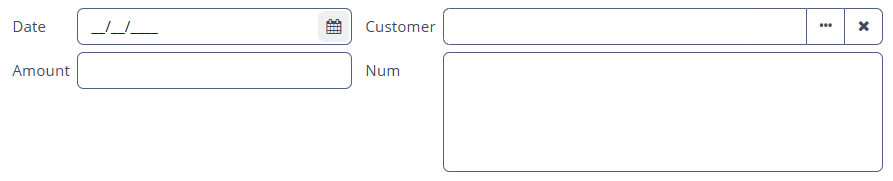
To add |
Column Attributes
-
id
– an optional column identifier, which allows you to refer to it in case of screen extension. -
width
– specifies the field width of a column. By default, fields have a width of200px
. In this attribute, the width can be specified both in pixels and in the percentage of the total horizontal width of the column. -
childrenCaptionWidth
– specifies fixed captions width for nested fields. Set-1
to use auto size. -
childrenCaptionAlignment
– defines the alignment of the nested fields captions. Two options are available:LEFT
andRIGHT
. The default value isLEFT
. Applicable only when thecaptionPosition
attribute isLEFT
.
All XML Attributes
align - caption - captionAsHtml - captionPosition - childrenCaptionAlignment - childrenCaptionWidth - colspan - contextHelpText - contextHelpTextHtmlEnabled - css - dataContainer - description - descriptionAsHtml - editable - enable - box.expandRatio - height - htmlSanitizerEnabled - icon - id - responsive - rowspan - stylename - visible - width