Image
Image
component is designed for displaying images from different sources. The component can be bound to a data container or configured programmatically.
Component’s XML-name: image
.
Basics
The Image
component can display the value of an entity attribute of FileRef
or byte[]
type. In the example below, the entity has the image
attribute which is a reference to a file in file storage:
@JmixEntity
@Table(name = "UIEX1_PERSON")
@Entity(name = "uiex1_Person")
public class Person {
/* other attributes */
@Column(name = "IMAGE", length = 1024)
private FileRef image;
In the simplest case, the component can be declaratively associated with data using the dataContainer and property attributes:
<data readOnly="true">
<instance id="personDc"
class="ui.ex1.entity.Person">
<fetchPlan extends="_base"/>
<loader/>
</instance>
</data>
<layout spacing="true">
<image id="fileRefImage"
dataContainer="personDc"
property="image"/>
</layout>
In the example above, the component displays the image
attribute of the Person
entity located in the personDc
data container.
Resources
Alternatively, the Image
component can display images from different resources. You can set the resource type declaratively using the nested XML elements described below, or programmatically using the setSource()
method.
To define |
ClasspathResource
A resource that is located in the classpath.
-
In XML:
classpath
element with thepath
attribute.For example, if your image is located at
ui/ex1/screen/component/image/jmix-logo.png
undersrc/main/resources
, you can specify the displayed resource as follows:<image scaleMode="SCALE_DOWN" width="20%" height="20%"> <resource> <classpath path="ui/ex1/screen/component/image/jmix-logo.png"/> </resource> </image>
-
In Java:
ClasspathResource
interface.image.setSource(ClasspathResource.class) .setPath("ui/ex1/screen/component/image/jmix-logo.png");
FileResource
A resource that is stored in the server file system.
-
In XML:
file
element with thepath
attribute.<image scaleMode="SCALE_DOWN" width="20%" height="20%"> <resource> <file path="D:\JMIX\jmix-logo.png"/> </resource> </image>
-
In Java:
FileResource
interface.image.setSource(FileResource.class) .setFile(file);
FileStorageResource
An image stored in the file storage.
-
In XML:
property
attribute ofimage
.<image id="fileRefImage" dataContainer="personDc" property="image"/>
-
In Java:
FileStorageResource
interface.image.setSource(FileStorageResource.class) .setFileReference(person.getImage());
RelativePathResource
An image served statically by the application.
By default, static content is served from /static
, /public
, /resources
, or /META-INF/resources
directories of the classpath (see details in the Spring Boot documentation).
-
In XML:
relativePath
element with thepath
attribute.For example, an image located at
src/main/resources/static/images/jmix-icon-login.svg
can be displayed as follows:<image> <resource> <relativePath path="images/jmix-icon-login.svg"/> </resource> </image>
The image will also be available at
http://{host}:{port}/images/jmix-icon-login.svg
. -
In Java:
RelativePathResource
interface.image.setSource(RelativePathResource.class) .setPath("images/jmix-icon-login.svg");
StreamResource
An image provided by an InputStream
.
-
In Java:
StreamResource
interface.byte[] picture; // picture = ... image.setSource(StreamResource.class) .setStreamSupplier(() -> new ByteArrayInputStream(picture));
ThemeResource
An image located in the current theme.
In the example below, an image is located in the images
directory of the helium-ext
custom theme:
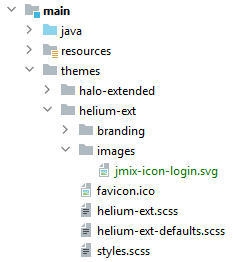
Then you can use it in the Image
component as follows:
-
In XML:
theme
element with thepath
attribute.<image> <resource> <theme path="images/jmix-icon-login.svg"/> </resource> </image>
-
In Java:
ThemeResource
interface:image.setSource(ThemeResource.class) .setPath("images/jmix-icon-login.svg");
UrlResource
An image that can be loaded from an arbitrary URL.
-
In XML:
url
element with theurl
attribute.<image scaleMode="SCALE_DOWN" width="20%" height="20%"> <resource> <url url="https://www.jmix.io/uploads/framework_image_9efadbc372.svg"/> </resource> </image>
-
In Java:
UrlResource
interface.try { image.setSource(UrlResource.class) .setUrl(new URL("https://www.jmix.io/images/jmix-logo.svg")); } catch (MalformedURLException e) { log.error("Invalid URL format", e); }
Resource Settings
-
bufferSize
- size of the download buffer in bytes used for this resource. -
cacheTime
- length of cache expiration time in milliseconds. -
mimeType
- MIME type of the resource.
For example:
<image>
<resource>
<url url="https://avatars3.githubusercontent.com/u/17548514?v=4&s=200"
mimeType="image/png"/>
</resource>
</image>
Attributes
-
alternateText
- sets an alternate text for an image in case the resource is not set or unavailable.
-
scaleMode
- applies the scale mode to the image. It corresponds to theobject-fit
CSS property. The following scale modes are available:-
FILL
- the image is stretched according to the size of the component. -
CONTAIN
- the image is compressed or stretched to fit the component dimensions while preserving the proportions. -
COVER
- the image is compressed or stretched to fill the entire component while preserving the proportions. If the image proportions do not match the component’s proportions then the image will be clipped to fit. -
SCALE_DOWN
- the image is sized as ifNONE
orCONTAIN
were specified, whichever would result in a smaller concrete object size. -
NONE
- the image is not resized.
-
Methods
To programmatically manage the Image
component, use the following methods:
-
setValueSource()
- sets the data container, and the entity attribute name. OnlyFileRef
andbyte[]
type of attributes are supported.The data container can be set programmatically, for example, to display images in table cells:
<data readOnly="true"> <collection id="personsDc" class="ui.ex1.entity.Person"> <fetchPlan extends="_base"/> <loader id="personsDl"> <query> <![CDATA[select e from uiex1_Person e]]> </query> </loader> </collection> </data> <layout spacing="true"> <groupTable id="personsTable" width="100%" dataContainer="personsDc"> <columns> <column id="name"/> </columns> </groupTable> </layout>
@Autowired private GroupTable<Person> personsTable; @Autowired private UiComponents uiComponents; @Subscribe public void onInit(InitEvent event) { personsTable.addGeneratedColumn("image", entity -> { Image<FileRef> image = uiComponents.create(Image.NAME); image.setValueSource( new ContainerValueSource<>( personsTable.getInstanceContainer(entity), "image")); image.setHeight("100px"); image.setScaleMode(Image.ScaleMode.CONTAIN); return image; }); }
-
setSource()
- sets the resource for the component. The method accepts the resource type and returns the resource object that can be configured using the fluent interface.<layout spacing="true"> <image id="programmaticImage"/> </layout>
@Autowired private Image<FileRef> programmaticImage; @Subscribe public void onInit(InitEvent event) { try { URL url = new URL("https://www.jmix.io/images/jmix-logo.svg"); programmaticImage.setSource(UrlResource.class).setUrl(url); } catch (MalformedURLException e) { log.error("Error", e); } }
Events and Handlers
To generate a handler stub in Jmix Studio, select the component in the screen descriptor XML or in the Jmix UI hierarchy panel and use the Handlers tab of the Jmix UI inspector panel. Alternatively, you can use the Generate Handler button in the top panel of the screen controller. |
ClickEvent
See ClickEvent.
SourceChangeEvent
SourceChangeEvent
is sent when a source of an image is changed. SourceChangeEvent
has the following methods:
-
getNewSource()
-
getOldSource()
@Subscribe("image")
public void onImageSourceChange(ResourceView.SourceChangeEvent event) {
notifications.create()
.withCaption("Old source was: " + event.getOldSource() +
"; new source is: " + event.getNewSource());
}
To register the event handler programmatically, use the addSourceChangeListener()
component method.
All XML Attributes
You can view and edit attributes applicable to the component using the Jmix UI inspector panel of the Studio’s Screen Designer. |
Image XML Attributes
align - alternateText - box.expandRatio - caption - captionAsHtml - colspan - contextHelpText - contextHelpTextHtmlEnabled - css - dataContainer - description - descriptionAsHtml - editable - enable - height - htmlSanitizerEnabled - icon - id - property - required - requiredMessage - property - required - requiredMessage - rowspan - scaleMode - stylename - visible - width
Resources XML Elements
classpath - file - relativePath - theme - url