PropertyFilter
PropertyFilter
is a component used for displaying a single filtering condition. The component is related to the entity property and can automatically render a proper layout for setting a condition value.
The component can be used inside the Filter component and independently.
Component’s XML-name: propertyFilter
.
Basics
In the general case, PropertyFilter
consists of a label with the entity property caption, operation label or selector (=
, contains
, in
, >
, etc.), and a field for editing a condition value.

The example of the propertyFilter
declaration is provided below.
<data>
<collection id="customersDc" class="ui.ex1.entity.Customer">
<fetchPlan extends="_base">
<property name="city" fetchPlan="_base"/>
</fetchPlan>
<loader id="customersDl">
<query>
<![CDATA[select e from uiex1_Customer e]]>
</query>
</loader>
</collection>
</data>
<layout spacing="true" expand="customersTable">
<propertyFilter property="age"
operation="GREATER_OR_EQUAL"
dataLoader="customersDl">
</propertyFilter>
</layout>
dataLoader
, property
, and operation
are required attributes.
-
dataLoader
sets a DataLoader related to the currentPropertyFilter
. -
property
contains the name of the entity attribute or properties path (for example,name
,order
,order.date
).You can use dynamic attributes, for example:
<propertyFilter property="+PassengerNumberofseats" operation="GREATER_OR_EQUAL" dataLoader="carsDl"/>
It is not necessary to have a dynamicAttributes facet on the screen.
Pay attention, if the dynamic attribute is an entity, then the entity’s internal attributes will not be available for filtering.
You can use multiple PropertyFilter
components in a screen. Components that have value are applied to the data loaders.
Condition Operations
operation
is a required attribute. It sets a filtering operation. The operation can be of the following types:
-
EQUAL
is suitable for string, numeric, date, reference attributes. Results include only entity instances where the data in the property column matches the condition value in the filter. -
NOT_EQUAL
is suitable for string, numeric, date, reference attributes. Results include only entity instances where the data in the property column does not match the condition value in the filter. -
GREATER
is suitable for numeric and date attributes. Results include only entity instances where the data in the property column is greater than the condition value in the filter. -
GREATER_OR_EQUAL
is suitable for numeric and date attributes. Results include only entity instances where the data in the property column is greater than or the same as the condition value in the filter. -
LESS
is suitable for numeric and date attributes. Results include only entity instances where the data in the property column is less than the condition value in the filter. -
LESS_OR_EQUAL
is suitable for numeric and date attributes. Results include only entity instances where the data in the property column is less than or the same as the condition value in the filter. -
CONTAINS
is suitable for string attributes. Results include only entity instances where the data in the property column has the condition value in the filter. -
NOT_CONTAINS
is suitable for string attributes. Results include only entity instances where the data in the property column does not contain the condition value in the filter. -
STARTS_WITH
is suitable for string attributes. Results include only entity instances where the data in the property column begins with the condition value in the filter. -
ENDS_WITH
is suitable for string attributes. Results include only entity instances where the data in the property column ends with the condition value in the filter. -
IS_SET
is suitable for string, numeric, date, reference attributes. The operator tests only the data in the property column that are not null. The ComboBox component, generated for this operation, displays two values:true
andfalse
. If the user selectstrue
, results include only entity instances where there is data in the column. Otherwise, results include only entity instances where there is no data in the column. -
IN_LIST
is suitable for string, numeric, date, reference attributes. Results include only entity instances where the data in the property column matches any list item. -
NOT_IN_LIST
is suitable for string, numeric, date, reference attributes. Results include only entity instances where the data in the property column does not match any list item. -
DATE_INTERVAL
is suitable for date attributes. Results include only entity instances where the data in the property column matches the values set in the Date interval editor.
PropertyFilter
can automatically determine and display a condition value component based on the type of the corresponding entity property and the operation. For example, for string and numeric attributes, the application uses TextField, for Date
- DateField, for enum values - ComboBox, for links to other entities - EntityPicker. For the IN_LIST
and NOT_IN_LIST
the application uses the ValuesPicker component.
PropertyFilter
also supports custom UI components for its value. You may describe UI component inside the propertyFilter
tag.
For example, you can use EntityComboBox instead of EntityPicker
:
<data>
<collection id="customersDc" class="ui.ex1.entity.Customer">
<fetchPlan extends="_base">
<property name="city" fetchPlan="_base"/>
</fetchPlan>
<loader id="customersDl">
<query>
<![CDATA[select e from uiex1_Customer e]]>
</query>
</loader>
</collection>
<collection id="citiesDc" class="ui.ex1.entity.City">
<fetchPlan extends="_base"/>
<loader id="citiesDl">
<query>
<![CDATA[select e from uiex1_City e]]>
</query>
</loader>
</collection>
</data>
<layout spacing="true" expand="customersTable">
<propertyFilter property="city" operation="EQUAL" dataLoader="customersDl">
<entityComboBox metaClass="uiex1_City" optionsContainer="citiesDc"/>
</propertyFilter>
</layout>
You can use EntityPicker
with custom lookup screen, for example:
<propertyFilter property="city" operation="EQUAL" dataLoader="customersDl">
<entityPicker metaClass="uiex1_City">
<actions>
<action id="entityLookup" type="entity_lookup">
<properties>
<property name="screenId" value="uiex1_City.browse"/>
<property name="openMode" value="DIALOG"/>
</properties>
</action>
</actions>
</entityPicker>
</propertyFilter>
The operationCaptionVisible
attribute defines the visibility of the operation caption. Possible values are true
and false
(true
by default).
Using the operationEditable
attribute, you can set whether an operation selector is visible. Possible values are true
and false
. The default value is false
. If you set operationEditable = true
, the operation field enables selecting the condition operator in run-time. The list of available operators depends on the attribute type.
Date Conditions
PropertyFilter
has the DATE_INTERVAL
operation. For this operation, the application uses the ValuePicker component with the custom action. It enables to open dialog to configure the date interval.
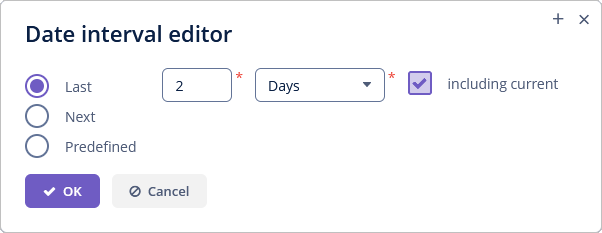
Automatic Applying
The autoApply
attribute sets whether the property filter should be automatically applied to the DataLoader when the condition value is changed. The default value is true
.
Managing Caption
You can set the custom caption of the property filter condition using the caption
attribute. If not specified, it is generated automatically based on the entity property caption and the operation caption (if the operationCaptionVisible is set to true
).
If you set the |
The captionPosition
attribute defines the filter caption position: TOP
or LEFT
. The default value is LEFT
.
The captionWidth
attribute specifies fixed caption width for PropertyFilter
. Set -1
to use auto size.
Default Value
You can set the default value for the filter condition in the defaultValue
attribute.
<propertyFilter property="age"
defaultValue="30"
operation="LESS_OR_EQUAL"
dataLoader="customersDl">
</propertyFilter>
Parameter Name
The parameterName
attribute sets the name of the associated query parameter name, used by condition. If not defined, then the parameter name is randomly generated.
Support for KeyValueCollectionContainer
PropertyFilter
can be bound to KeyValueCollectionContainer
, for example:
<data>
<keyValueCollection id="salesDc">
<loader id="salesLoader">
<query>
<![CDATA[select o.customer, o.customer.firstName,
sum(o.amount) from uiex1_Order o group by o.customer]]>
</query>
</loader>
<properties>
<property class="ui.ex1.entity.Customer" name="customerEntity"/>
<property datatype="string" name="customerName"/>
<property datatype="decimal" name="sum"/>
</properties>
</keyValueCollection>
</data>
<layout spacing="true" expand="customersTable">
<propertyFilter property="customerEntity.firstName" operation="STARTS_WITH"
dataLoader="salesLoader" operationEditable="true"/>
<propertyFilter property="customerName" operation="CONTAINS"
dataLoader="salesLoader" operationEditable="true"/>
</layout>
PropertyFilter
does not support key-value property that contains aggregate functions (COUNT
, SUM
, AVG
, MIN
, MAX
).
Events and Handlers
To generate a handler stub in Jmix Studio, select the component in the screen descriptor XML or in the Jmix UI hierarchy panel and use the Handlers tab of the Jmix UI inspector panel. Alternatively, you can use the Generate Handler button in the top panel of the screen controller. |
OperationChangeEvent
The OperationChangeEvent
is sent when the operation
property is changed. Example of subscribing to the event for a propertyFilter
defined in the screen XML with hobbyFilter
id:
@Subscribe("hobbyFilter")
public void onHobbyFilterOperationChange(PropertyFilter.OperationChangeEvent event) {
notifications.create()
.withCaption("Before: " + event.getPreviousOperation() +
". After: " + event.getNewOperation())
.show();
}
Programmatic registration of the event handler: use the addOperationChangeListener()
component method.
ValueChangeEvent
ValueChangeEvent
is sent on the value changes when the user finished the value input, for example, after the Enter press or when the component loses focus. ValueChangeEvent
has the following methods:
-
getPrevValue()
returns the value of the component before the change. -
getValue()
method return the current value of the component.
Example of subscribing to the event for a propertyFilter
defined in the screen XML with hobbyFilter
id:
@Subscribe("hobbyFilter")
public void onHobbyFilterValueChange(HasValue.ValueChangeEvent event) {
notifications.create()
.withCaption("Before: " + event.getPrevValue() +
". After: " + event.getValue())
.show();
}
Programmatic registration of the event handler: use the addValueChangeListener()
component method.
All XML Attributes
You can view and edit attributes applicable to the component using the Jmix UI inspector panel of the Studio’s Screen Designer. |
align - autoApply - caption - captionAsHtml - captionPosition - captionWidth - colspan - contextHelpText - contextHelpTextHtmlEnabled - css - dataLoader - defaultValue - description - descriptionAsHtml - editable - enable - box.expandRatio - height - htmlSanitizerEnabled - icon - id - operation - operationCaptionVisible - operationEditable - parameterName - property - required - requiredMessage - responsive - rowspan - stylename - tabIndex - visible - width