Using URL History and Navigation API
This section contains examples of using the URL History and Navigation API.
Suppose we have the Event
entity and the EventInfo
screen with information about the selected event.
The EventInfo
screen controller contains the @Route
annotation to specify the route to the screen:
@UiController("eventInfo")
@UiDescriptor("event-info.xml")
@Route("event-info")
public class EventInfo extends Screen {
}
As a result, a user can open the screen by entering http://localhost:8081/#main/event-info
in the address bar:
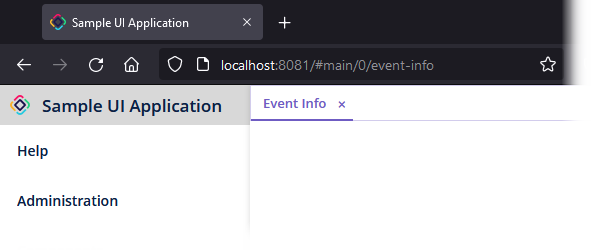
When the screen is opened, the address also contains a state mark.
Mapping State to URL
Suppose that the EventInfo
screen shows information about a single event at a time, and it has controls to switch the selected events. You may want to reflect the currently viewed event in the URL to copy the URL and later open the screen for this particular event just by pasting the URL to the address bar.
The following code implements mapping of the selected event to the URL:
@UiController("eventInfo")
@UiDescriptor("event-info.xml")
@Route("event-info")
public class EventInfo extends Screen {
@Autowired
protected EntityComboBox<Event> eventField;
@Autowired
protected UrlRouting urlRouting;
@Subscribe("selectBtn")
protected void onSelectBtnClick(Button.ClickEvent e) {
Event event = eventField.getValue(); (1)
if (event == null){
urlRouting.replaceState(this); (2)
return;
}
String serializedEventId = UrlIdSerializer.serializeId(event.getId()); (3)
urlRouting.replaceState(this, ImmutableMap.of("event_id", serializedEventId)); (4)
}
}
1 | Get the current event from EntityComboBox . |
2 | Remove URL parameters if no event is selected. |
3 | Serialize event’s id with the UrlIdSerializer helper. |
4 | Replace the current URL state with the new one containing serialized event id as a parameter. |
As a result, the application URL is changed when the user selects an event and clicks the Select Event button:
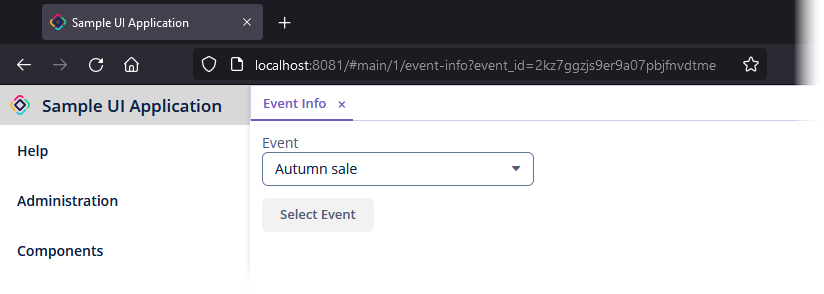
UrlParamsChangedEvent
Now let’s implement the last requirement: when a user enters the URL with the route and the event_id
parameter, the application must show the screen with the corresponding event selected.
Below is an example of subscribing to UrlParamsChangedEvent
in the screen controller code:
@Subscribe
protected void onUrlParamsChanged(UrlParamsChangedEvent event) {
String serializedEventId = event.getParams().get("event_id"); (1)
UUID eventId = (UUID) UrlIdSerializer.deserializeId(UUID.class, serializedEventId); (2)
eventField.setValue(dataManager.load(Event.class).id(eventId).one()); (3)
}
1 | Get the parameter value from UrlParamsChangedEvent . |
2 | Deserialize the event id . |
3 | Load the event instance and set it to the field. |