FileMultiUploadField
The FileMultiUploadField
component allows you to upload files to the temporary storage. After that, you can process them as File
objects in the server file system or move to the file storage.
When you click on a button, a standard OS file picker window appears, where you can select multiple files for upload.
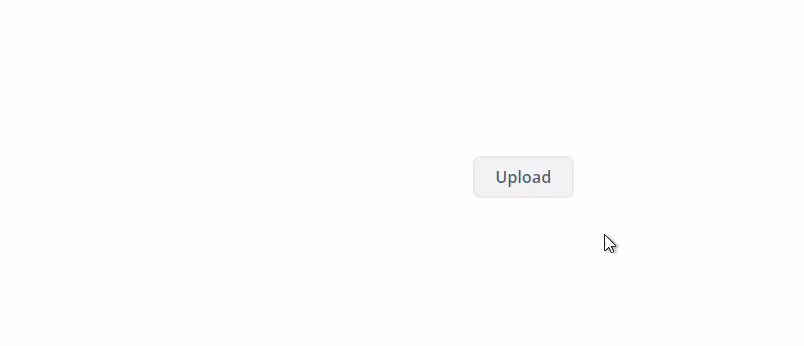
Component’s XML-name: fileMultiUpload
.
Basics
Usage example:
Declare the component in the XML screen descriptor:
<fileMultiUpload id="fileMultiUploadField"
caption="Upload"/>
(1)
@Autowired
private FileMultiUploadField fileMultiUploadField;
@Autowired
private TemporaryStorage temporaryStorage;
@Autowired
private Notifications notifications;
@Subscribe
public void onInit(InitEvent event) {
(2)
fileMultiUploadField.addQueueUploadCompleteListener(queueUploadCompleteEvent -> { (3)
for (Map.Entry<UUID, String> entry : fileMultiUploadField.getUploadsMap().entrySet()) { (4)
UUID fileId = entry.getKey();
String fileName = entry.getValue();
FileRef fileRef = temporaryStorage.putFileIntoStorage(fileId, fileName); (5)
}
notifications.create()
.withCaption("Uploaded files: " + fileMultiUploadField.getUploadsMap().values())
.show();
fileMultiUploadField.clearUploads(); (6)
});
fileMultiUploadField.addFileUploadErrorListener(queueFileUploadErrorEvent ->
notifications.create()
.withCaption("File upload error")
.show());
}
1 | In the screen controller, inject the component itself and the TemporaryStorage interface. |
2 | On screen initialization, add listeners which will react to successful uploads and errors. |
3 | The component uploads all selected files to the temporary storage and invokes the listener added by the addQueueUploadCompleteListener() method. |
4 | In this listener, invoke the FileMultiUploadField.getUploadsMap() method to obtain a map of temporary storage file identifiers to file names. |
5 | Then you can transfer uploaded files to the permanent file storage and get corresponding FileRef objects. |
6 | After processing, you should clear the list of files by calling the clearUploads() method to prepare for further uploads. |
Listeners
The FileMultiUploadField
component has the following listeners, described in the FileStorageUploadField
component:
Attributes
The FileMultiUploadField
component has the following attributes, described in the FileStorageUploadField
component:
Methods
-
getUploadsMap()
- returnsMap
of file ids inTemporaryStorage
to file names. -
clearUploads()
- clears the list of uploaded files.
All XML Attributes
accept - box.expandRatio - caption - captionAsHtml - colspan - contextHelpText - contextHelpTextHtmlEnabled - description - descriptionAsHtml - dropZone - dropZonePrompt - enable - css - fileSizeLimit - height - htmlSanitizerEnabled - icon - id - pasteZone - permittedExtensions - responsive - rowspan - stylename - tabindex - visible - width