PasswordField
This is a text field that displays echo characters instead of those entered by a user.
Component’s XML-name: passwordField
.
Basics
The PasswordField
is similar to TextField
apart from the ability to set datatype
. PasswordField
is intended to work with text and entity attributes of type String
only.
An example of the PasswordField
:
<passwordField id="passwordField"
caption="Secret password field"/>
@Autowired
private PasswordField passwordField;
@Autowired
private Notifications notifications;
@Subscribe("showPasswordBtn")
protected void onShowPasswordBtnClick(Button.ClickEvent event) {
notifications.create()
.withCaption(passwordField.getValue())
.show();
}
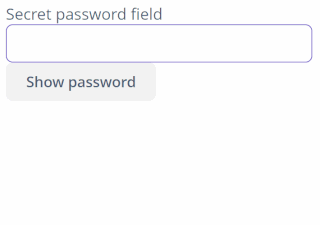
Attributes
-
The
autocomplete
attribute allows you to enable saving passwords in the web browser. It is disabled by default. -
The
capsLockIndicator
attribute allows you to set theid
of aCapsLockIndicator
component that should indicate Caps Lock state for thispasswordField
.
Validation
To check values entered into the PasswordField
component, you can use a validator in a nested validators
element.
The following predefined validators are available for PasswordField
:
In the example below, we will show a SizeValidator
usage for passwordValidField
:
<passwordField id="passwordValidField"
caption="Secret password field">
<validators>
<size max="10" min="4"/>
</validators>
</passwordField>
Events and Handlers
To generate a handler stub in Jmix Studio, select the component in the screen descriptor XML or in the Jmix UI hierarchy panel and use the Handlers tab of the Jmix UI inspector panel. Alternatively, you can use the Generate Handler button in the top panel of the screen controller. |
Validator
Adds a validator instance to the component. The validator
must throw ValidationException
if the value is not valid.
If you are not satisfied with the predefined validators, add your own validator instance:
@Install(to = "zipField", subject = "validator")
protected void zipFieldValidator(Integer value) {
if (value != null && String.valueOf(value).length() != 6)
throw new ValidationException("Zip must be of 6 digits length");
}
ValueChangeEvent
See ValueChangeEvent.
PasswordField XML Attributes
You can view and edit attributes applicable to the component using the Jmix UI inspector panel of the Studio’s Screen Designer. |
align - autocomplete - buffered - capsLockIndicator - caption - captionAsHtml - colspan - contextHelpText - contextHelpTextHtmlEnabled - css - dataContainer - description - descriptionAsHtml - editable - enable - box.expandRatio - height - htmlSanitizerEnabled - htmlName - icon - id - inputPrompt - maxLength - property - required - requiredMessage - responsive - rowspan - stylename - tabIndex - textChangeEventMode - visible - width