UI Components
The WebDAV add-on includes UI components that facilitate working with WebDAV documents.
To add these components on the view, use Jmix Studio.
Click Add Component in the actions panel, then find type webdav
in the search field. From the list of results, select the desired component and double-click on it.
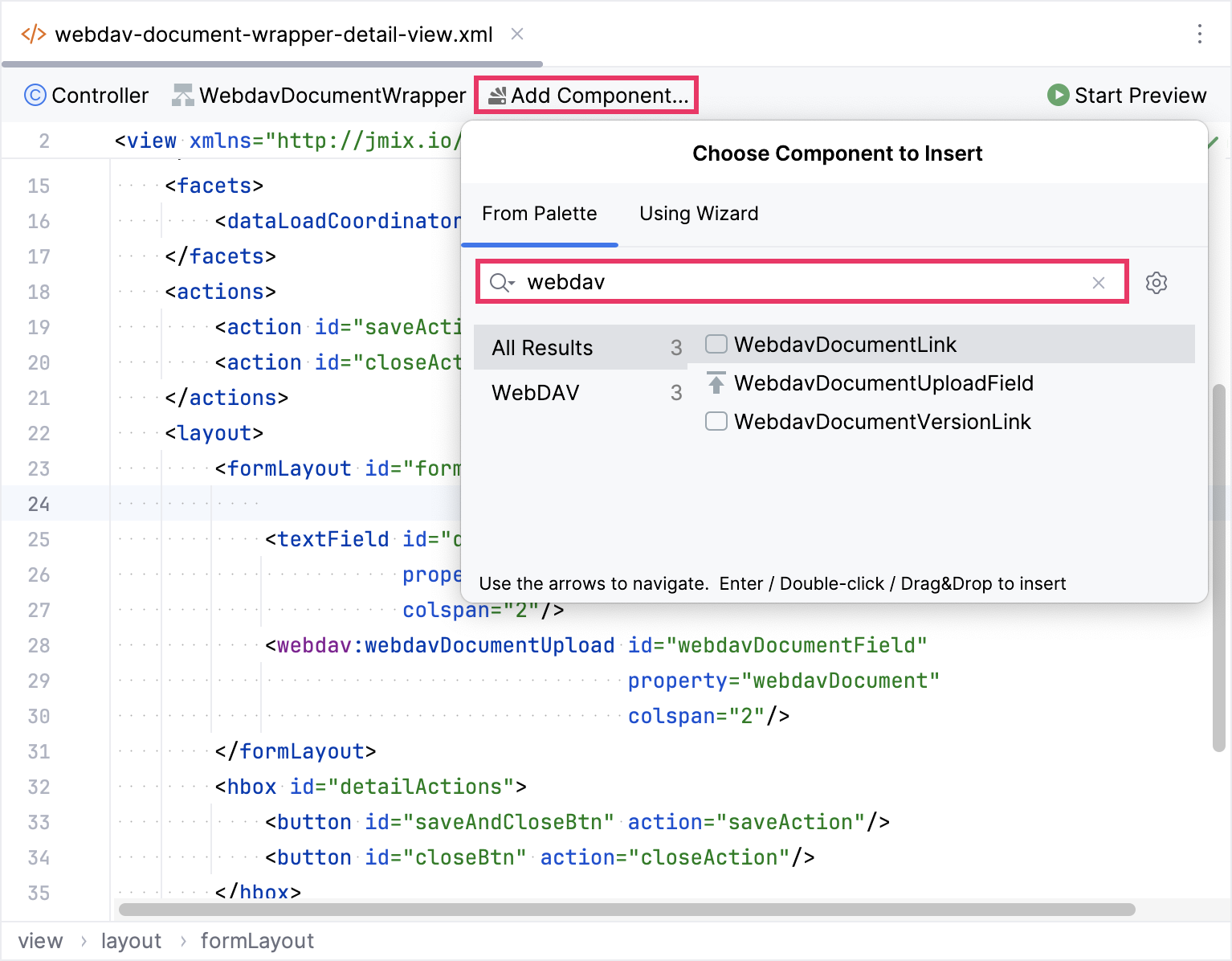
The new element will be added in both the Jmix UI structure panel and in the XML. You can configure attributes like id, height, width, etc., in the same way as it is done for other UI components.
<webdav:webdavDocumentUpload id="webdavDocumentField"
property="webdavDocument"
colspan="2"/>
If you don’t use the view designer, declare the webdav
namespace in your view’s XML descriptor manually:
<view xmlns="http://jmix.io/schema/flowui/view"
xmlns:webdav="http://jmix.io/schema/webdav/ui"
title="msg://webdavDocumentWrapperDetailView.title"
focusComponent="form">
You can inject the UI component into the controller by utilizing the Inject to Controller action in the Jmix UI structure panel:
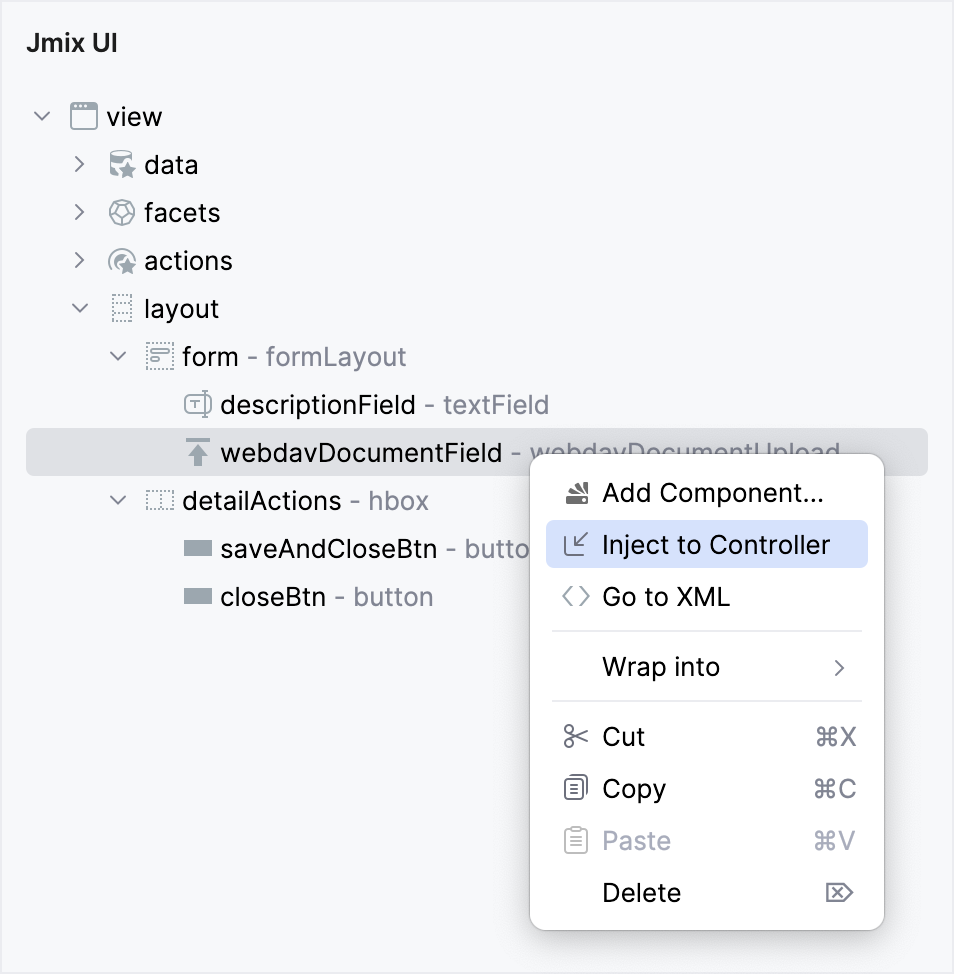
Alternatively, you can employ the Inject button available in the actions panel:
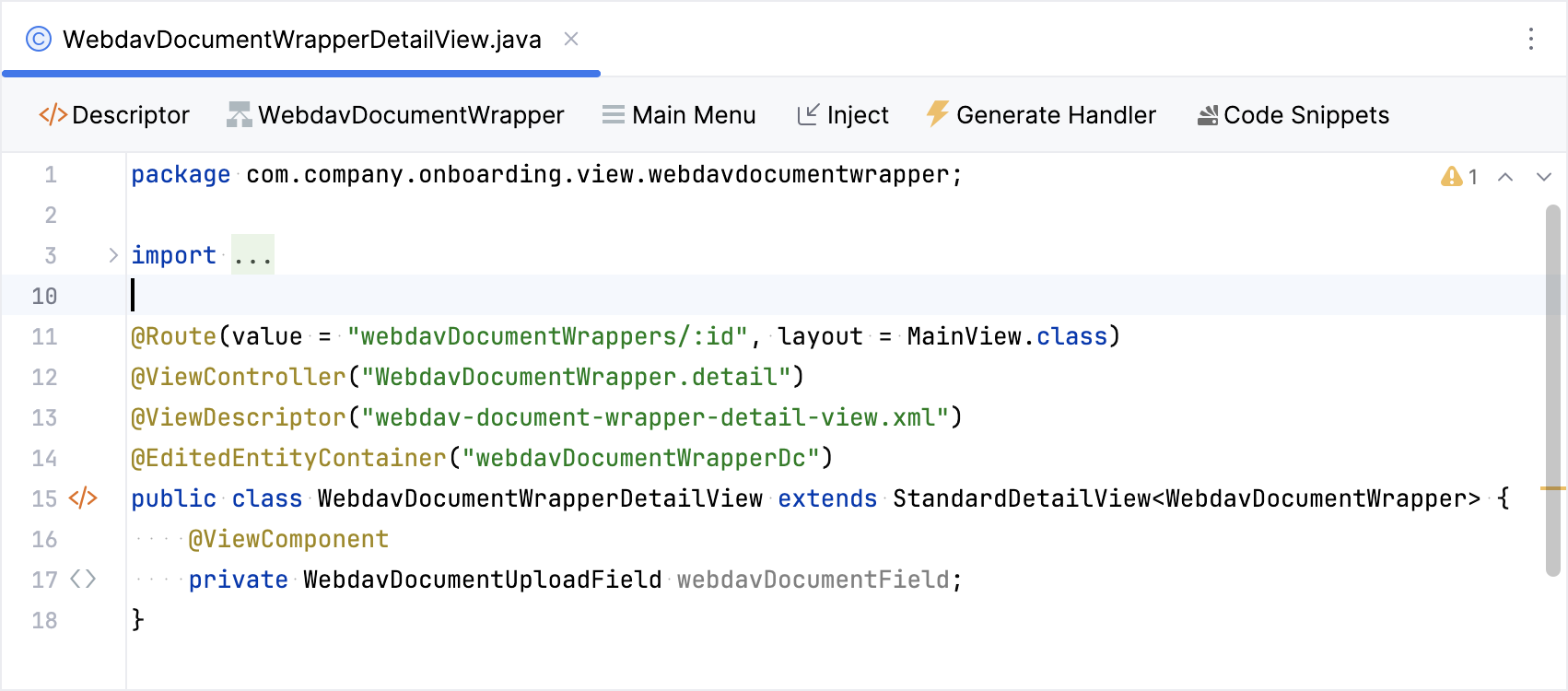
For the component to be injectable into the controller, it must have the id attribute specified.
|
Now you can interact with the webDAV UI component programmatically by accessing its methods directly:
@ViewComponent
private WebdavDocumentUploadField webdavDocumentField;
@Subscribe
public void onInit(final InitEvent event) {
webdavDocumentField.setMaxFileSize(5242880);
}
WebdavDocumentLink
WebdavDocumentLink
is a UI component enabling users to open documents in desktop office applications. Additionally, if document versioning is supported, users can view all versions of the document.
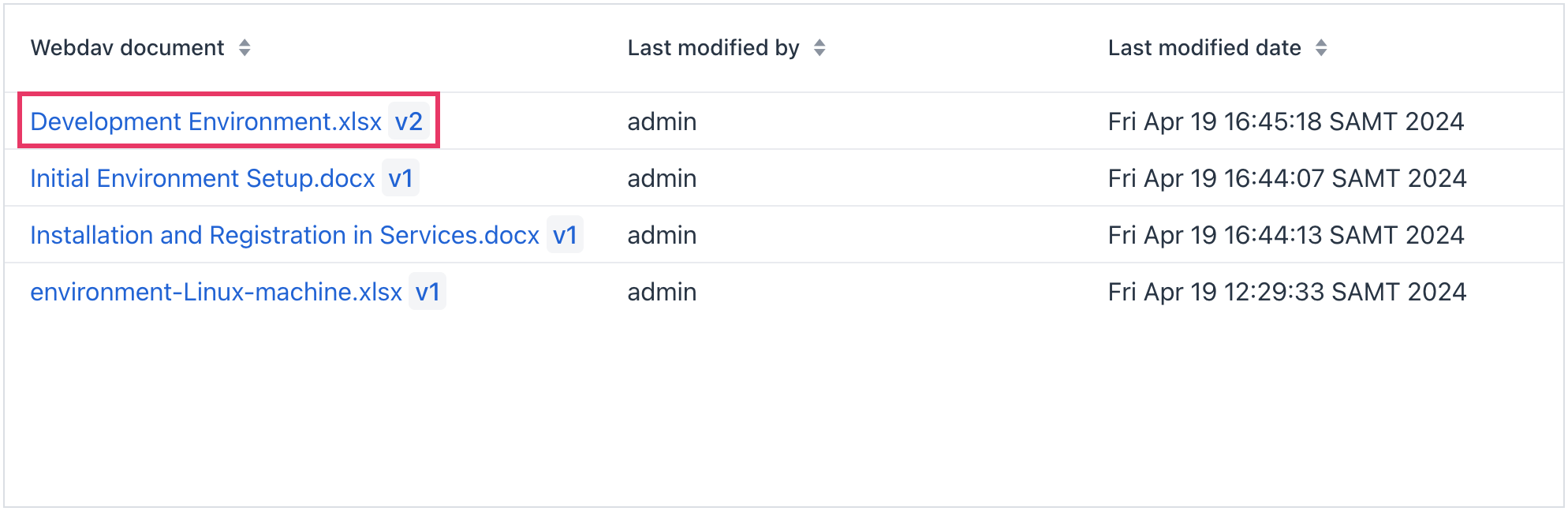
The component offers the following features:
-
Opening the most recent version of a document for viewing and editing.
-
Opening previous versions of a document in read-only mode (for further information, refer to WebDAV Document Versions).
In the example below, WebdavDocumentLink
will be rendered in the left column of the data grid:
<dataGrid id="webdavDocumentWrappersDataGrid"
width="100%"
minHeight="20em"
dataContainer="webdavDocumentWrappersDc"
columnReorderingAllowed="true">
<columns resizable="true">
<column property="webdavDocument"/>
<column property="description"/>
<column property="lastModifiedBy"/>
<column property="lastModifiedDate"/>
</columns>
</dataGrid>
The renderer
handler creates and returns the WebdavDocumentLink
component:
@Supply(to = "webdavDocumentWrappersDataGrid.webdavDocument", subject = "renderer")
private Renderer<WebdavDocumentWrapper> webdavDocumentWrappersDataGridWebdavDocumentRenderer() {
return new ComponentRenderer<>( (1)
() -> uiComponents.create(WebdavDocumentLink.class), (2)
(link, wrapper) -> {
WebdavDocument webdavDocument = wrapper.getWebdavDocument();
if (webdavDocument != null) {
link.setWebdavDocument(webdavDocument);
}
});
}
1 | The method returns a Renderer object that creates a UI component to be rendered in the column. |
2 | The WebdavDocumentLink component instance is created using the UiComponents factory. |
WebdavDocumentVersionLink
WebdavDocumentVersionLink
allows opening a specific document version in desktop office applications in read-only mode. The component shows the file name of a particular document version.
To create WebdavDocumentVersionLink
, use the UiComponents
factory.
WebdavDocumentVersionLink webdavDocumentVersionLink =
uiComponents.create(WebdavDocumentVersionLink.class);
webdavDocumentVersionLink.setWebdavDocumentVersion(documentVersion);
WebdavDocumentUploadField
The WebdavDocumentUploadField
component is specifically designed for working with documents.
The XML component name: webdavDocumentUpload
.
The component provides the following functionalities:
-
Uploading a file to create a new document or a new version of an existing document.
-
Opening a document for viewing or editing.
-
Downloading the latest or previous versions of a document.
-
Creating new versions of a document based on existing versions.
Work Modes
The component operates in two modes:
-
Versioning Enabled: When versioning is enabled, the
WebdavDocumentUploadField
component is displayed without any limitations. -
Versioning Disabled: When versioning is disabled, the
WebdavDocumentUploadField
component does not display a link to the most recent version of the document.