simplePagination
simplePagination
is a component used to load data by pages. It is designed to be used together with dataGrid, and treeDataGrid components.
-
XML element:
simplePagination
-
Java class:
SimplePagination
Basics
simplePagination
has a simple view with a count of rows and navigation buttons.
It can also have a drop-down list for the number of items per page.
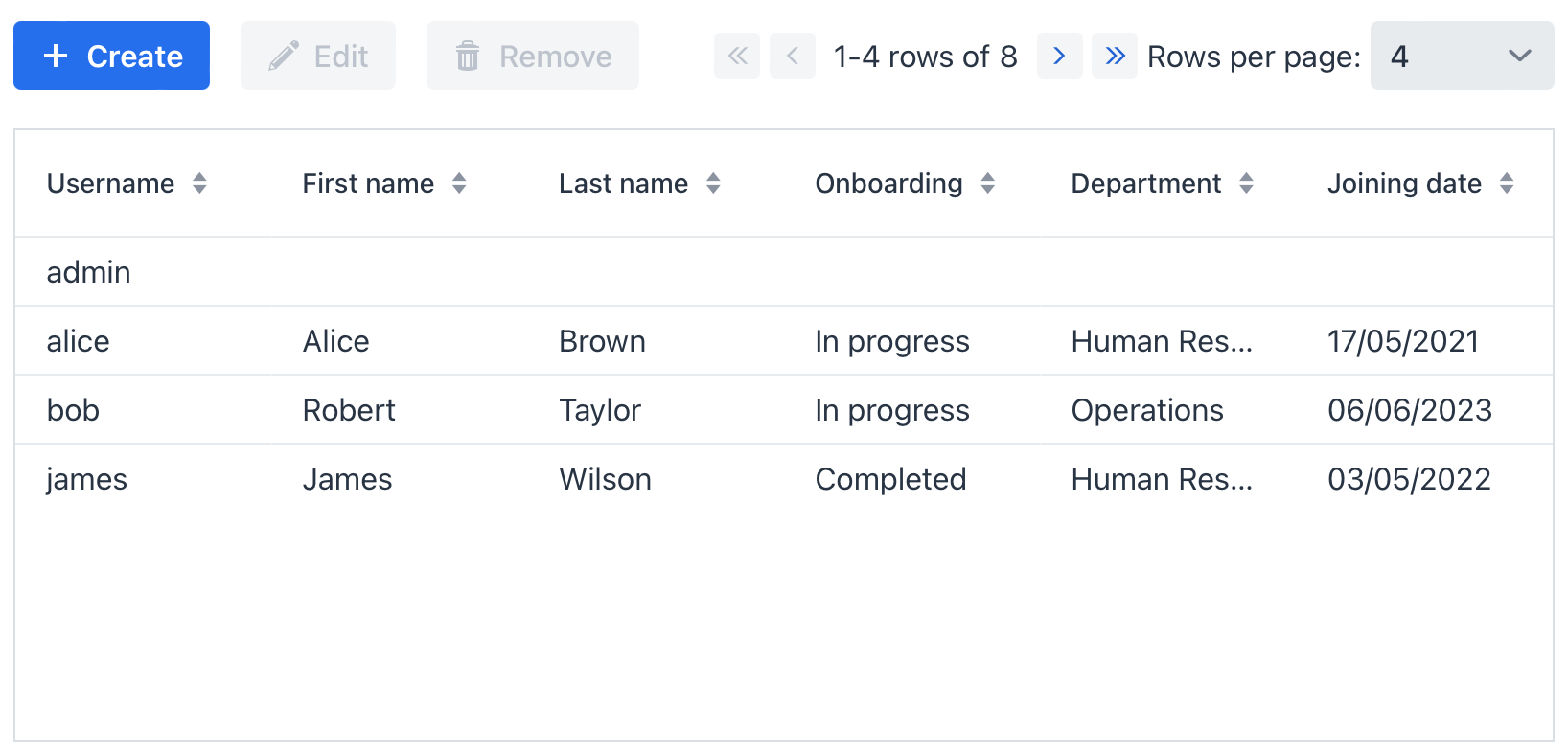
By default, Jmix Studio generates simplePagination
when creating StandardListView for an entity.
<data readOnly="true">
<collection id="usersDc"
class="com.company.onboarding.entity.User">
<fetchPlan extends="_base">
<property name="department" fetchPlan="_base"/>
</fetchPlan>
<loader id="usersDl">
<query>
<![CDATA[select e from User e order by e.username]]> (1)
</query>
</loader>
</collection>
</data>
<layout expand="usersTable">
<simplePagination itemsPerPageVisible="true"
id="simplePagination"
dataLoader="usersDl"/> (2)
</layout>
1 | Define a collection loader to load User entities. |
2 | Connect simplePagination to data using the dataLoader attribute. |
Items per Page
simplePagination
has a special comboBox
with options to limit the number of items for one page. To make it visible, set the itemsPerPageVisible
attribute to the true
value. The default value is false
.
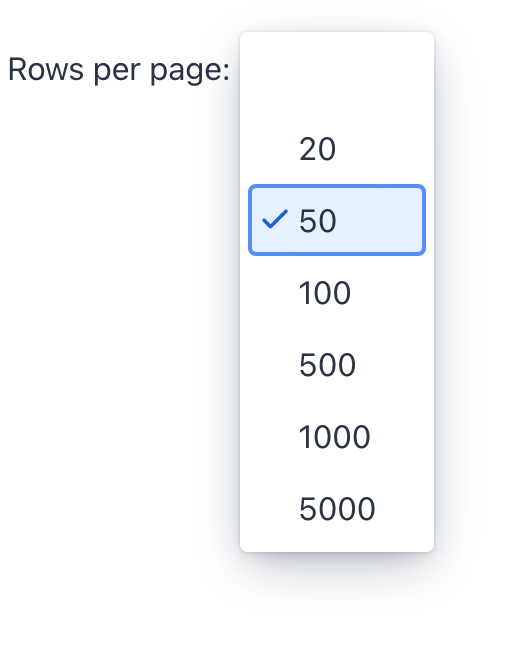
The default value of this list is specified in the jmix.flowui.component.pagination-items-per-page-items property.
You can configure a custom list of options using the itemsPerPageItems
attribute. The value of the attribute should be a comma-separated list of options:
<simplePagination dataLoader="usersDl"
itemsPerPageVisible="true"
itemsPerPageItems="2, 4, 6"/>
Options that are less than or equal to 0
are ignored. Options that are greater than entity’s maximum fetch size are replaced by that maximum value.
Use the itemsPerPageDefaultValue
attribute to set a default value from the list of options:
<simplePagination dataLoader="usersDl"
itemsPerPageVisible="true"
itemsPerPageItems="2, 4, 6"
itemsPerPageDefaultValue="4"/>
The itemsPerPageUnlimitedItemVisible
attribute sets the visibility of unlimited (null) option value in the items per page comboBox
. The default value is true
.
When the null option is selected in the comboBox
, the component will try to load all data it can with the current maximum fetch size limitation.
The maximum fetch size for all entities is defined by jmix.flowui.default-max-fetch-size UI property. Its default value is 10000. A particular entity may have different maximum fetch size, set with jmix.flowui.entity-max-fetch-size. |
Attributes
Handlers
To generate a handler stub in Jmix Studio, use the Handlers tab of the Jmix UI inspector panel or the Generate Handler action available in the top panel of the view class and through the Code → Generate menu (Alt+Insert / Cmd+N). |
AfterRefreshEvent
io.jmix.flowui.component.PaginationComponent.AfterRefreshEvent
is fired after data refresh.
BeforeRefreshEvent
io.jmix.flowui.component.PaginationComponent.BeforeRefreshEvent
is fired before refreshing the data when the user clicks next, previous, etc. You can prevent the data container refresh by invoking the preventRefresh()
method, for example:
@Subscribe("simplePagination")
public void onSimplePaginationBeforeRefresh(
final PaginationComponent.BeforeRefreshEvent<SimplePagination> event) {
if (Objects.requireNonNull(event.getSource().getPaginationLoader()).size() > 10) (1)
event.preventRefresh(); (2)
}
1 | Check the number of instances in the data loader. |
2 | Prevent refreshing the data. |
totalCountDelegate
Sets delegate which is used to get the total count of items. For example:
@Autowired
private DataManager dataManager;
@Install(to = "simplePagination", subject = "totalCountDelegate")
private Integer simplePaginationTotalCountDelegate(final LoadContext<User> loadContext) {
return dataManager.loadValue("select count(e) from User e", Integer.class).one();
}