3. Email Generation from Templates
Let’s walk through an example of generating an email from subject and message body templates created in the previous section.
Before we begin, confirm that the Email Sending add-on is installed. If it is not currently installed, please install it before continuing.
Next, configure the required email sending parameters to enable successful email delivery.
Adding Notify by Email Button
Let’s add a Notify by Email button to the booking list view. This button will allow users to send an email notification to the creator of the booking selected in the data grid.
Find booking-list-view.xml
in the Jmix tool window and double-click it. The view designer appears. Select actions
within the Jmix UI hierarchy panel or in the XML descriptor. Next click the Add button in the component inspector panel. From the drop-down list, choose Action:
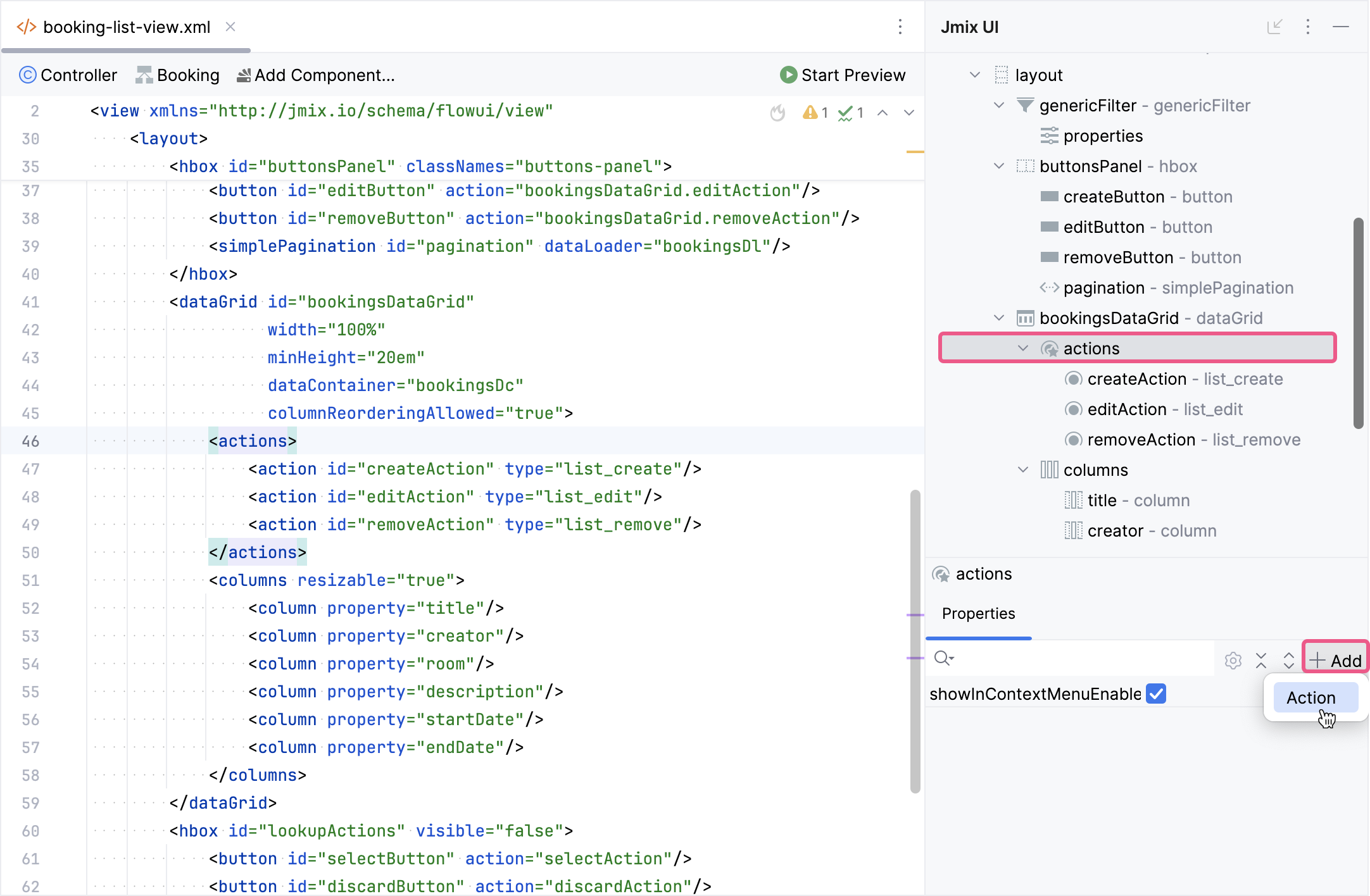
Select the list_itemTracking
action in the Add Action dialog. The list_itemTracking
action will automatically become active only when a specific item is selected in the associated dataGrid
.
Configure the following attributes for this action:
<action id="notifyEmail"
type="list_itemTracking"
icon="ENVELOPE"
text="msg://notifyEmail.text"/>
Then, in the buttonsPanel
hbox, add a button and associate it with the newly created action.
<hbox id="buttonsPanel" classNames="buttons-panel">
<!--...-->
<button id="notifyEmailButton" action="bookingsDataGrid.notifyEmail"/>
</hbox>
Generating an Email from the Template
Generate an ActionPerformedEvent
handler method for the notifyEmailButton
action. Add the logic to it:
@ViewComponent
private DataGrid<Booking> bookingsDataGrid;
@Autowired
private MessageTemplatesGenerator messageTemplatesGenerator; (1)
@Autowired
private Emailer emailer; (2)
@Autowired
private Notifications notifications; (3)
@Subscribe("bookingsDataGrid.notifyEmail")
public void onBookingsDataGridNotifyEmail(final ActionPerformedEvent event) {
Booking booking = bookingsDataGrid.getSingleSelectedItem();
User creator = booking.getCreator(); (4)
String email = creator.getEmail();
if (email == null) {
showNoEmailNotification(creator); (5)
return;
}
List<String> messages = messageTemplatesGenerator.generateMultiTemplate()
.withTemplateCodes("booking-email-subject", "booking-email-body") (6)
.withParams(
Map.of(
"booking", booking,
"today", new Date()
)) (7)
.generate();
EmailInfo emailInfo = EmailInfoBuilder.create()
.setAddresses(email)
.setSubject(messages.get(0))
.setBody(messages.get(1))
.setBodyContentType("text/html; charset=UTF-8")
.build(); (8)
try {
emailer.sendEmail(emailInfo); (9)
} catch (EmailException e) {
showSendingErrorNotification(email);
}
showSendingSuccessNotification(email); (10)
}
private void showSendingErrorNotification(String email) {
notifications.create("Failed to send email to %s".formatted(email))
.withThemeVariant(NotificationVariant.LUMO_ERROR)
.show();
}
private void showNoEmailNotification(User creator) {
notifications.create("%s did not specify an email".formatted(creator.getDisplayName()))
.withThemeVariant(NotificationVariant.LUMO_ERROR)
.show();
}
private void showSendingSuccessNotification(String email) {
notifications.create("The message has been successfully sent to %s".formatted(email))
.withThemeVariant(NotificationVariant.LUMO_SUCCESS)
.show();
}
1 | A bean for generating messages from templates. |
2 | A bean for sending emails. |
3 | A bean for displaying notifications to the user. |
4 | Fetches the selected booking and its creator from the bookingsDataGrid . |
5 | Checks if the creator has an email address. If not, a notification is displayed, and the method exits. |
6 | Generates the message subject and body using FreeMarker templates (booking-email-subject and booking-email-body ). |
7 | Passes dynamic parameters (booking , today ) to the templates. |
8 | Constructs an EmailInfo object with the recipient’s email, subject, body, and content type. |
9 | Attempts to send the email using the emailer bean. If an error occurs, a failure notification is displayed. |
10 | Displays a success notification if the email is sent successfully. |
Email Sending and Viewing
Let’s verify the functionality of the template-based email generation process. This test ensures that booking details are accurately populated into the message template and delivered to the intended recipient.
-
Launch the application and navigate to the Application → Bookings view.
-
Create a new booking instance by clicking the Create button. When creating the booking, make sure to select an existing user with a valid email address from the creator selection dropdown. This email address will be used to receive the generated email. Fill in the other required fields with relevant test data.
-
In the bookings data grid, locate and select the booking you just created. Once selected, click the Notify by Email button. This action will trigger the email generation and sending process.
-
The application should display a notification indicating that the email has been successfully sent.
-
After observing the successful notification, open your email client and check the inbox of the user whose email address was used for the booking. Verify that the email was received and that the content of the email is accurately populated from the booking details and the pre-configured message template.
Summary
In this section, you learned how to work with message templates to automate email notifications within your app.
You gained practical experience in adding the Notify by Email button to a view, configuring an action to trigger the email sending process, and writing the code to dynamically generate an email message using data from the selected entity.
You also learned the importance of configuring email sending parameters and ensuring the Email Sending add-on is installed.