Maps UI Component
The GeoMap
UI component displays a map in the screens. You can define the general map parameters along with the layers in the component’s XML descriptor.
To enable using the component in the screen, declare the maps
namespace in the root element in the screen XML descriptor:
<window xmlns="http://jmix.io/schema/ui/window"
xmlns:maps="http://jmix.io/schema/maps/ui"
caption="msg://salespersonEdit.caption">
An example of defining a geoMap
XML element with a layers
element containing one raster layer and two vector layers:
<maps:geoMap id="map"
height="400px"
width="800px"
centerX="-99.755859"
centerY="39.164141"
zoom="4"> (1)
<maps:layers selectedLayer="salespersonLayer"> (2)
<maps:tile id="tileLayer"
urlPattern="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png"
attribution="© <a href="https://www.openstreetmap.org/copyright">
OpenStreetMap</a> contributors"/> (3)
<maps:vector id="territoryLayer" dataContainer="territoryDc"/> (4)
<maps:vector id="salespersonLayer"
dataContainer="salespersonDc"
editable="true"/> (5)
</maps:layers>
</maps:geoMap>
1 | Parameters define the window size, coordinates of the initial geographical center of the map — longitude and latitude , and the initial zoom level. |
2 | Sets the selected layer - a layer the map is focused on. |
3 | Specifies a tile layer. |
4 | Specifies non-editable vector layer. |
5 | Specifies editable vector layer. |
Here how it performs in the application.
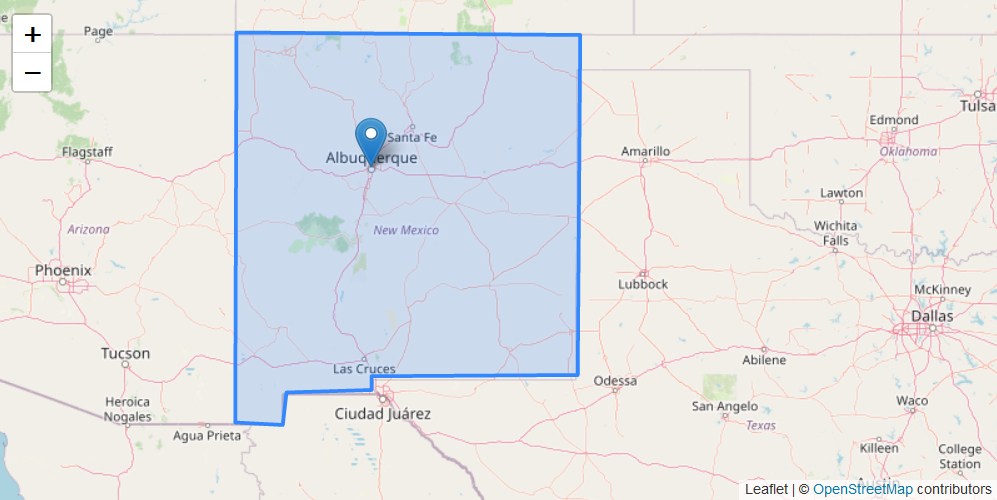
Additional configuration of the map and its layers can be performed in the screen controller. You need to add the component declared in the XML descriptor with @Autowired
annotation:
@Autowired
private GeoMap map;
@Autowired
private InstanceContainer<Territory> territoryDc;
@Autowired
private InstanceContainer<Salesperson> salespersonDc;
@Subscribe
protected void onBeforeShow(BeforeShowEvent event) {
map.setHeight("400px");
map.setWidth("800px");
map.setCenter(-99.755859D, 39.164141D);
map.setZoomLevel(4);
TileLayer tileLayer = new TileLayer("tileLayer");
tileLayer.setUrl("https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png");
tileLayer.setAttributionString("© <a href=\"http://www.openstreetmap.org/copyright\"" +
">OpenStreetMap</a> © <a href=\"https://carto.com/attributions\">CARTO</a>");
map.addLayer(tileLayer);
VectorLayer<Territory> territoryLayer = new VectorLayer<>("territoryLayer", territoryDc);
map.addLayer(territoryLayer);
VectorLayer<Salesperson> salespersonLayer = new VectorLayer<>("salespersonLayer", salespersonDc);
salespersonLayer.setEditable(true);
map.addLayer(salespersonLayer);
map.selectLayer(salespersonLayer);
}
Was this page helpful?
Thank you for your feedback