Configuring Charts
Each chart type has its own set of attributes and methods, which replicate the functionality of the corresponding charts from the AmCharts library. Details of the properties and methods of the charts are available in the documentation.
Any configuration attribute can be set to null. In this case, the system will use the default value (except the cases specified in the AmCharts documentation).
Messages used for displaying charts can be overridden or localized in the message bandle.
Declarative Configuration
The elements described below can be used for declarative configuration of all types of charts.
allLabels
This element contains the label
elements with the label text and its properties. Labels can be placed on a certain position on the chart, for example:
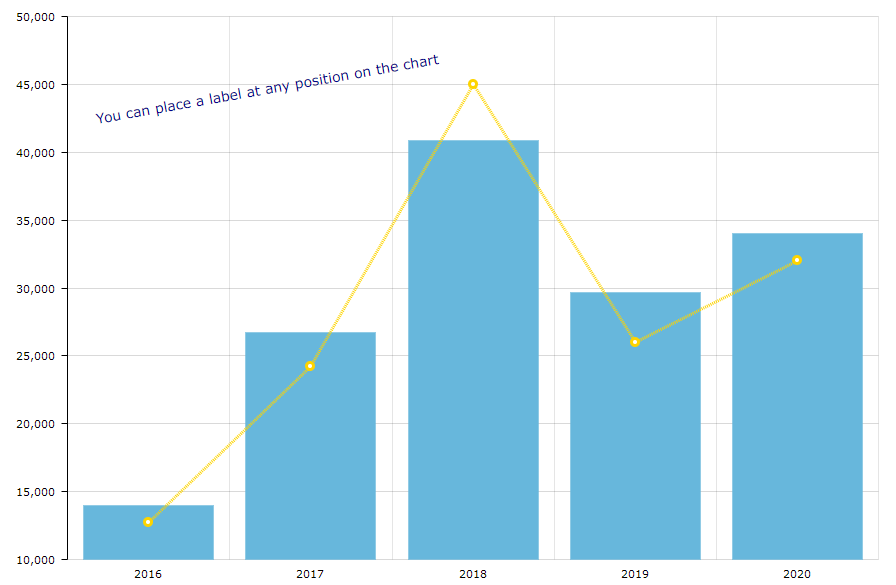
<chart:allLabels>
<chart:label x="300"
y="1000"
text="You can place a label at any position on the chart"
color="DARKBLUE"
rotation="-10"
align="CENTER"
size="14"/>
</chart:allLabels>
balloon
This element sets the configurations of balloons (tooltips) of the chart that follow the mouse cursor when you roll-over the data items. For example:
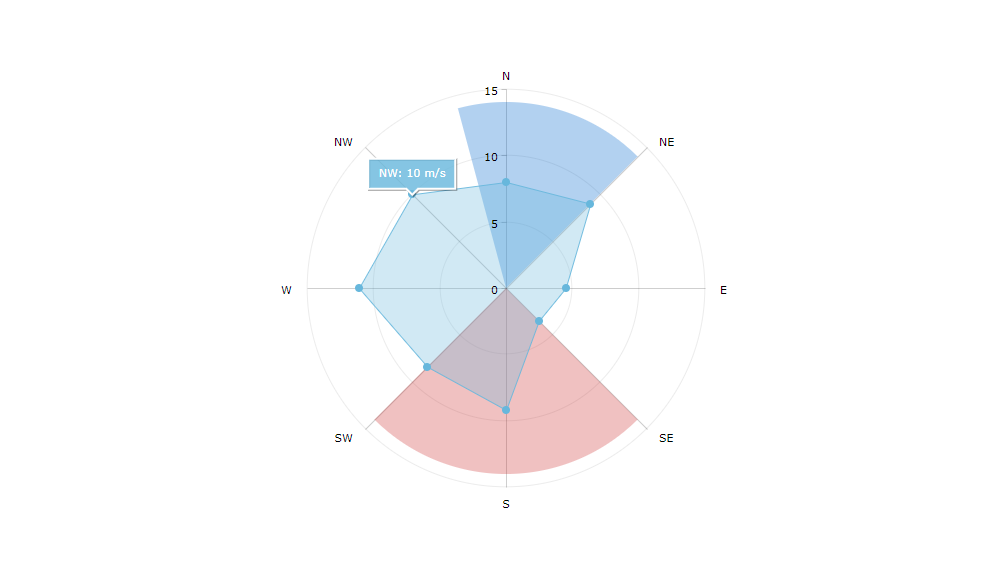
<chart:graphs>
<chart:graph balloonText="[[category]]: [[value]] m/s"
bullet="ROUND"
fillAlphas="0.3"
valueField="value"/>
</chart:graphs>
<chart:balloon adjustBorderColor="false"
color="WHITE"
horizontalPadding="10"
verticalPadding="8"/>
The balloon text is defined by the balloonText
attribute of each chart graph.
Interpolation of chart data is available for all fields used in the chart, such as titleField
, valueField
, category
, value
, description
, percents
, open
, and so on, as well as HTML tags.
You can also fetch more entity attributes from the data provider using the additionalFields
attribute. This attribute allows you to add the passed entity attributes to the chart query and send the extracted data to the UI so that you can use the entity attribute names directly in the chart configuration.
In the example below, title
is the graph title, category
is the value on the category axis, value
is the value on the value axis, and additional
is the IncomeExpenses
entity attribute fetched to be interpolated in the balloonText
:
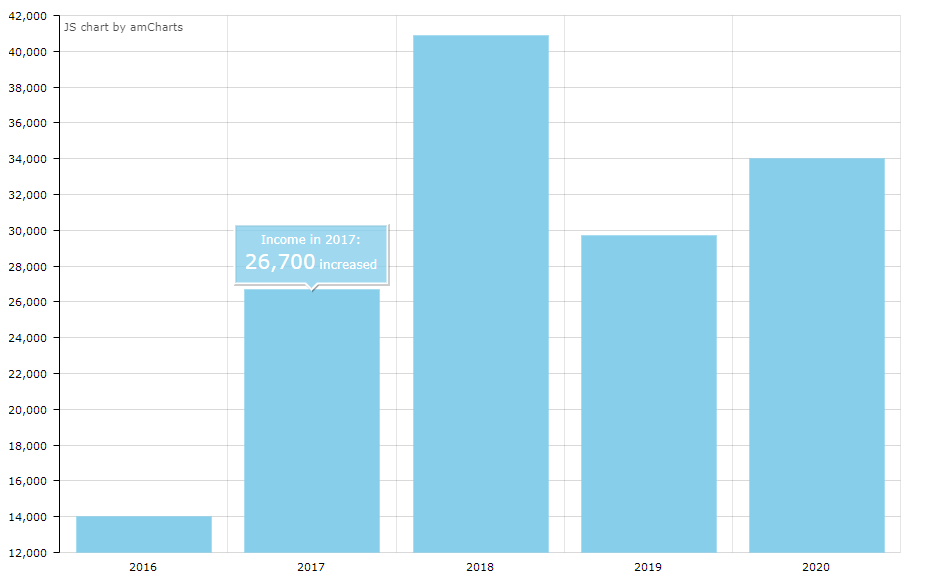
<chart:serialChart additionalFields="additional"
addClassNames="true"
autoMargins="false"
categoryField="year"
dataContainer="incomeExpensesDc"
height="100%"
marginLeft="50"
marginRight="10"
marginTop="10"
startDuration="1"
theme="LIGHT"
width="90%">
<chart:balloon adjustBorderColor="false"
color="WHITE"
horizontalPadding="10"
verticalPadding="8"/>
<chart:graphs>
<chart:graph alphaField="alpha"
balloonText="<span style='font-size:12px;'>[[title]] in [[category]]:<br>
<span style='font-size:20px;'>[[value]]</span> [[additional]]</span>"
dashLengthField="dashLengthColumn"
title="Income"
type="COLUMN"
valueField="income"/>
</chart:graphs>
You can add a list of fields declaratively as a comma-separated string:
<chart:serialChart id="serialChart"
additionalFields="income,year"
<...>
/>
<...>
</chart:serialChart>
or programmatically in the screen controller:
@Autowired
private SerialChart serialChart;
@Subscribe
public void onInit(InitEvent event) {
List<String> fields = Arrays.asList("income", "year");
serialChart.setAdditionalFields(fields);
}
chartScrollbar
This element is available for SerialChart and XYChart.
-
You can set the concrete graph to zoom by the scrollbar, for example:
chart:chartScrollbar<chart:chartScrollbar autoGridCount="true" backgroundAlpha="0" color="#AAAAAA" graph="g1" offset="30" oppositeAxis="false"/>
-
GanttChart
can have achart:valueScrollbar
element for scrolling the value axis, whilechart:chartScrollbar
will be used for zooming the category axis.chart:valueScrollbar<chart:valueScrollbar autoGridCount="true" color="BLACK"/> <chart:chartScrollbar autoGridCount="true" color="DARKOLIVEGREEN"/>
cursor
An optional element adding a cursor to the chart. The cursor follows the mouse pointer and shows a tooltip with the value of the corresponding point on a chart.
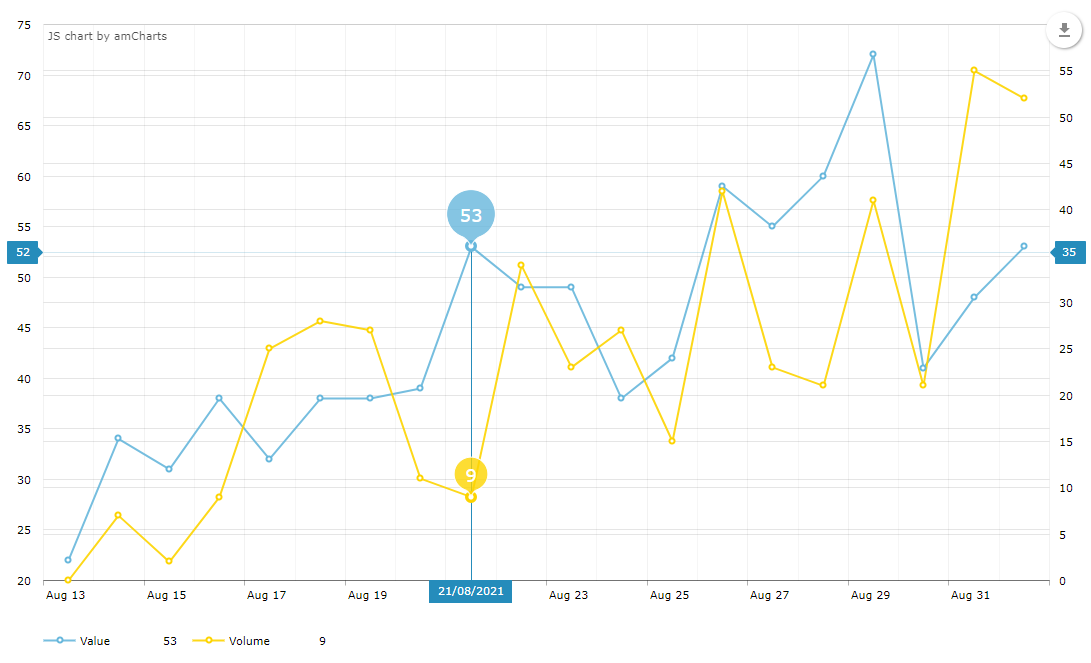
<chart:chartCursor cursorAlpha="1"
cursorColor="#258cbb"
cursorPosition="MOUSE"
limitToGraph="g1"
pan="true"
valueLineAlpha="0.2"
valueLineBalloonEnabled="true"
valueLineEnabled="true"
valueZoomable="true"/>
data
This is an optional element for data binding used mostly for prototyping.
export
This is an optional element that enables chart export. The default export implementation adds a floating Download button on the chart:
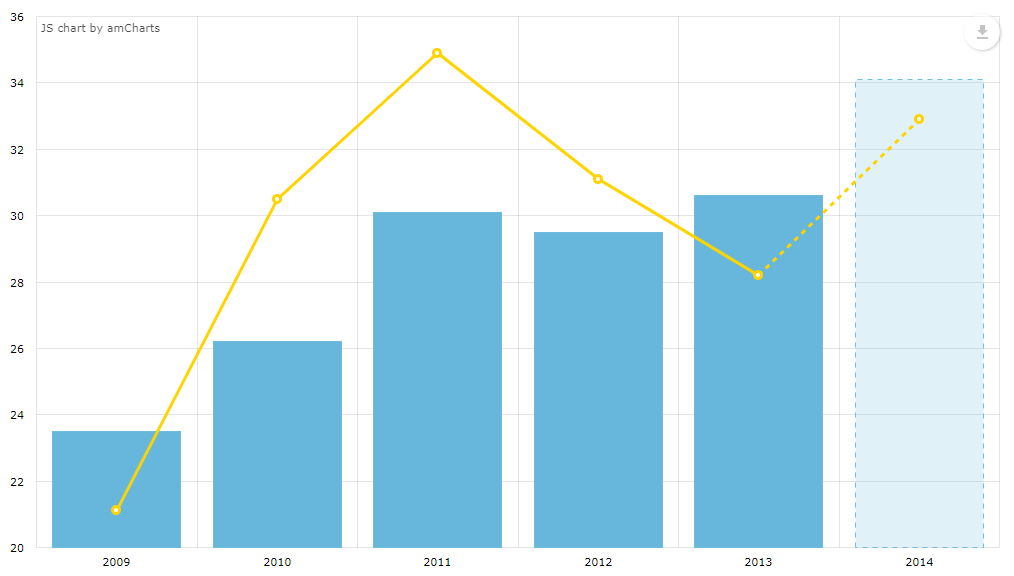
guides
This element add horizontal/vertical guidelines.
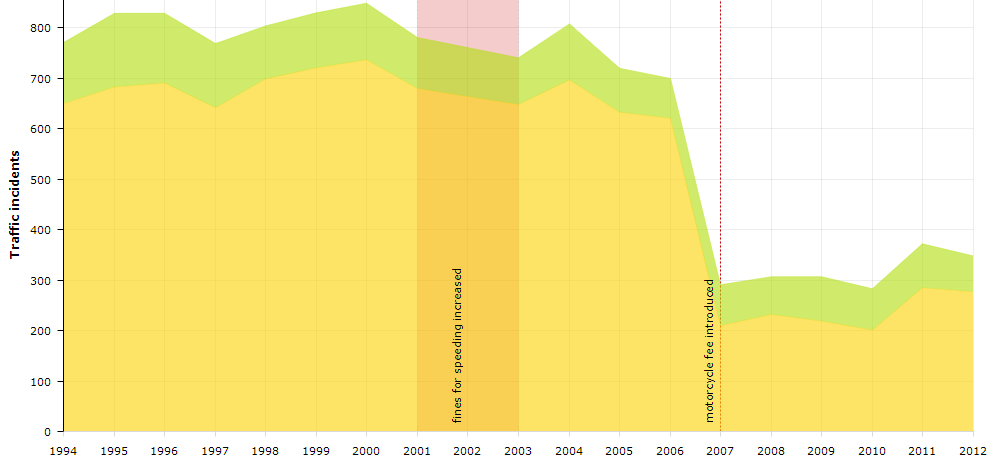
<chart:guides>
<chart:guide category="2001"
dashLength="2"
fillAlpha="0.2"
fillColor="#CC0000"
inside="true"
label="fines for speeding increased"
labelRotation="90"
toCategory="2003"/>
<chart:guide category="2007"
dashLength="2"
inside="true"
label="motorcycle fee introduced"
labelRotation="90"
lineAlpha="1"
lineColor="#CC0000"/>
</chart:guides>
legend
This element defines the chart legend, for example:
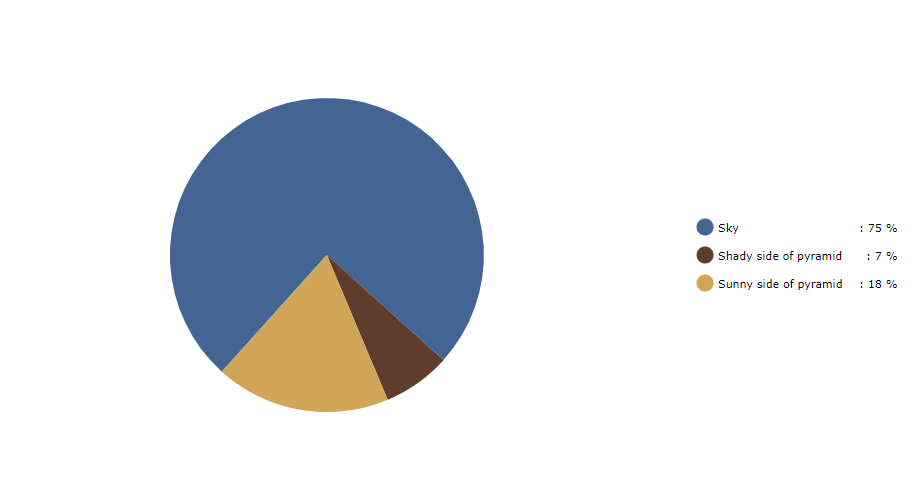
<chart:guides>
<chart:guide category="2001"
dashLength="2"
fillAlpha="0.2"
fillColor="#CC0000"
inside="true"
label="fines for speeding increased"
labelRotation="90"
toCategory="2003"/>
<chart:guide category="2007"
dashLength="2"
inside="true"
label="motorcycle fee introduced"
labelRotation="90"
lineAlpha="1"
lineColor="#CC0000"/>
</chart:guides>
nativeJson
JSON configuration of the chart.
titles
This element add axis titles on the chart.
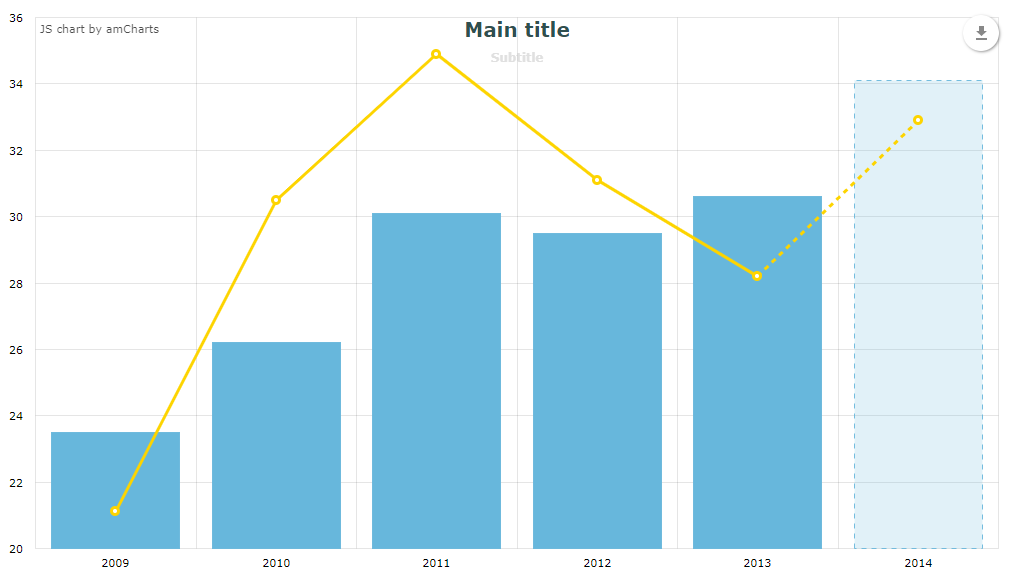
<chart:titles>
<chart:title alpha="1"
bold="true"
color="DARKSLATEGREY"
size="20"
tabIndex="0"
text="Main title"/>
<chart:title alpha="0.5"
color="SILVER"
size="12"
text="Subtitle"/>
</chart:titles>
responsive
This is a chart plugin that makes the chart responsive.
It enables scaling-down and -up chart visual features automatically in order to adjust the chart to the screen resolution changes. You can find more information on the responsive plugin on the AmCharts website.
The responsive
element should contain the enclosed rules element where the rules of accommodation are defined. You can make the chart hide or show the legend, axis titles, guides, chart titles, zoom controls, move labels inside plot area and so on:
<chart:responsive enabled="true">
<chart:rules>
<chart:rule maxWidth="400">
<![CDATA[
{
"precision": 2,
"legend": {
"enabled": false
},
"valueAxes": {
"inside": true
}
}
]]>
</chart:rule>
</chart:rules>
</chart:responsive>
Programmatic Configuration
Configuring charts in a screen controller is performed following the same logic. You can configure a simple property, as well as a composite object set:
@Autowired
private PieChart pieChart;
@Subscribe
public void onInit(InitEvent event) {
pieChart.setWidth("700px");
pieChart.setTitleField("description")
.setValueField("value")
.setStartAngle(312)
.setLegend(new Legend()
.setMarkerType(MarkerType.CIRCLE)
.setPosition(LegendPosition.RIGHT)
.setMarginRight(80))
.addLabels(
new Label()
.setText("Sample Chart")
.setSize(26)
.setBold(true)
.setAlign(Align.CENTER),
new Label()
.setText("extra information")
.setAlign(Align.RIGHT))
.setLabelTickColor(Color.GOLDENROD)
.setColors(Arrays.asList(
Color.valueOf("#446493"),
Color.valueOf("#5E3D2C"),
Color.valueOf("#D0A557")))
.setDataProvider(initDataProvider());
}
private DataProvider initDataProvider() {
ListDataProvider dataProvider = new ListDataProvider();
dataProvider.addItem(new MapDataItem(
ImmutableMap.of("value", 75, "description", "Sky")));
dataProvider.addItem(new MapDataItem(
ImmutableMap.of("value", 7, "description", "Shady side of pyramid")));
dataProvider.addItem(new MapDataItem(
ImmutableMap.of("value", 18, "description", "Sunny side of pyramid")));
return dataProvider;
}