UI Components
WebdavDocumentLink
WebdavDocumentLink
is a UI component that allows users to open documents in desktop office applications. Also, if versioning is supported for a document, a user can see all versions of a document.
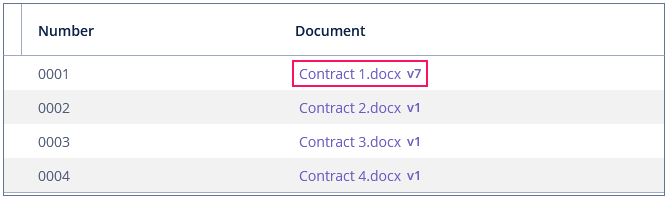
The component has the following functionality:
-
Opening the latest version of a document for viewing and editing.
-
Opening previous document versions in read-only mode (for more details, see WebDAV Document Versions).
In the example below, WebdavDocumentLink
is created for the docLink
generated column of the table component:
<groupTable id="contractsTable"
width="100%"
dataContainer="contractsDc">
<columns>
<column id="number"/>
<column id="docLink"
caption="Document"/>
</columns>
</groupTable>
The columnGenerator
handler creates and returns the WebdavDocumentLink
component:
@Autowired
protected UiComponents uiComponents;
@Install(to = "contractsTable.docLink", subject = "columnGenerator")
protected Component contractsTableDocLinkColumnGenerator(Contract contract) {
WebdavDocumentLink link = uiComponents.create(WebdavDocumentLink.class)
.withWebdavDocument(contract.getDocument()); (1)
link.setIsShowVersion(false); (2)
return link;
}
1 | The withWebdavDocument() method accepts the document instance for which to generate the link. |
2 | Using the setIsShowVersion() method, you can manage the version link visibility. By default, the vesrion link is shown if versioning is enabled for the document. |
WebdavDocumentVersionLink
WebdavDocumentVersionLink
enables opening a particular document version in desktop office applications in the read-only mode. The component displays a file name of a certain document version.
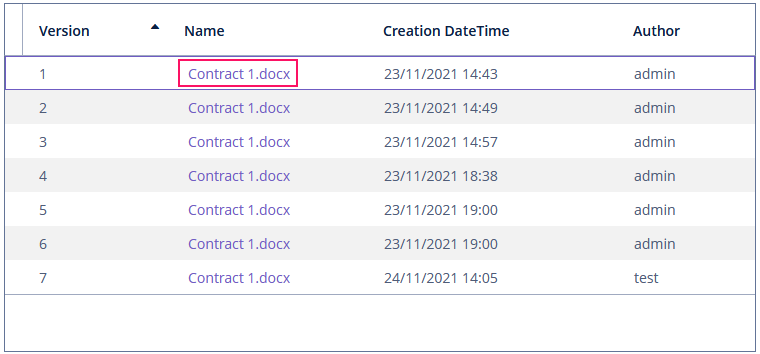
To create WebdavDocumentVersionLink
, use the UiComponents
factory.
WebdavDocumentVersionLink webdavDocumentVersionLink =
uiComponents.create(WebdavDocumentVersionLink.class);
webdavDocumentVersionLink.setWebdavDocumentVersion(documentVersion);
WebdavDocumentUploadField
WebdavDocumentUploadField
is designed to work with documents.
Component XML name: webdavDocumentUpload
.
The component has the following functionality:
-
Uploading a file to create a new document or a document version.
-
Opening a document for viewing or editing.
-
Downloading the latest or preceding document versions.
-
Creating new document versions based on previous ones.
Work Modes
The component works in the following two modes:
-
If versioning is enabled,
WebdavDocumentUploadField
is displayed without any restrictions. -
If versioning is disabled,
WebdavDocumentUploadField
does not show a link to the latest document version.
Declarative Creation
To declare the WebdavDocumentUploadField
component in a screen XML descriptor, first add a namespace with the http://jmix.io/schema/webdav/ui
URI to it. Then use this namespace with the component element, for example:
<window xmlns="http://jmix.io/schema/ui/window"
xmlns:webdav="http://jmix.io/schema/webdav/ui"
caption="msg://contractEdit.caption"
focusComponent="form">
<layout spacing="true" expand="editActions">
<form id="form" dataContainer="contractDc">
<column width="350px">
<webdav:webdavDocumentUpload id="documentField"
property="document"/>
</column>
</form>
</layout>
</window>
WebdavDocumentUploadField
extends SingleFileUploadField
and inherits all its properties:
accept - align - box.expandRatio - buffered - caption - captionAsHtml - clearButtonCaption - clearButtonDescription - clearButtonIcon - colspan - contextHelpText - contextHelpTextEnabled - css - dataContainer - description - descriptionAsHtml - dropZone - dropZonePrompt - editable - enable - fileSizeLimit fileStorage - height - htmlSanitizerEnabled - icon - id - pasteZone permittedExtensions - property - required - requiredMessage - responsive - rowspan - showClearButton - showFileName stylename - tabIndex - uploadButtonCaption - uploadButtonDescription - uploadButtonIcon - visible - width
Programmatic Usage
WebdavDocumentUploadField
has the API similar to FileStorageUploadField.
Using FileMultiUploadField
The below example shows how to create WebDAV documents from files uploaded using FileMultiUploadField:
@Autowired
protected FileMultiUploadField fileMultiUploadFieldVersioned;
@Autowired
protected TemporaryStorage temporaryStorage;
@Autowired
protected WebdavDocumentsManagementService webdavDocumentsManagementService;
@Autowired
protected Notifications notifications;
@Subscribe
protected void onInit(InitEvent event) {
fileMultiUploadFieldVersioned.addQueueUploadCompleteListener(e -> {
for (Map.Entry<UUID, String> entry :
fileMultiUploadFieldVersioned.getUploadsMap().entrySet()) {
UUID fileId = entry.getKey();
String fileName = entry.getValue();
FileRef fileRef = temporaryStorage.putFileIntoStorage(fileId, fileName); (1)
webdavDocumentsManagementService.createVersioningDocumentByFileRef(fileRef); (2)
}
notifications
.create()
.withType(Notifications.NotificationType.HUMANIZED)
.withCaption("Uploaded files: " +
fileMultiUploadFieldVersioned.getUploadsMap().values())
.show();
fileMultiUploadFieldVersioned.clearUploads();
});
fileMultiUploadFieldVersioned.addFileUploadErrorListener(e ->
notifications
.create()
.withCaption("File upload error")
.withType(Notifications.NotificationType.HUMANIZED)
.show());
}
1 | Save file to the file storage. |
2 | Create and save WebdavDocument . |