Export Actions
ExportAction
is a base list action for export table content with a defined exporter. An instance of TableExporter is required for this action.
The action should be defined for a list component (Table, GroupTable, TreeTable, DataGrid, TreeDataGrid, Tree).
ExportAction
has methods for managing functions that get value from the Table
or DataGrid
column.
-
addColumnValueProvider()
adds a function to get value from the column. -
removeColumnValueProvider()
removes a column value provider function by columnid
.
ExportAction
has two modes for export: all rows and selected rows. If no rows are selected, all rows will be exported by default without confirmation.
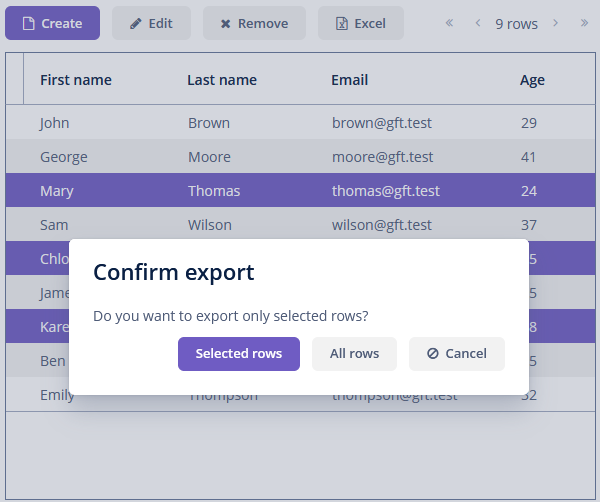
You can override the title and message of the dialog by adding messages with actions.exportSelectedTitle
and actions.exportSelectedCaption
keys to your message bundle.
Custom Export Action
You can define a custom action inherited from ExportAction
.
An example of creating a custom export action:
@ActionType(CustomExportAction.ID)
public class CustomExportAction extends ExportAction {
public static final String ID = "myExcelExport";
public CustomExportAction(String id) {
this(id, null);
}
public CustomExportAction() {
this(ID);
}
public CustomExportAction(String id, String shortcut) {
super(id, shortcut);
}
@Override
public void setApplicationContext(ApplicationContext applicationContext) {
super.setApplicationContext(applicationContext);
withExporter(CustomExporter.class); (1)
}
@Override
public String getIcon() {
return "font-icon:CHECK";
}
}
1 | If needed, you can use a custom table exporter. |
Now you can use the action in the screen controller:
@Autowired
protected Table<Customer> customersTable;
@Autowired
protected Button customBtn;
@Autowired
protected ApplicationContext applicationContext;
@Autowired
protected Actions actions;
@Subscribe
protected void onInit(InitEvent event) {
CustomExportAction customAction = actions.create(CustomExportAction.class);
customAction.setTableExporter(applicationContext.getBean(CustomExporter.class));
customAction.setTarget(customersTable);
customAction.setApplicationContext(applicationContext);
customBtn.setAction(customAction);
}
ExcelExportAction
ExcelExportAction
is an action extending ExportAction and designed to export the table content in XLSX format.
The action is implemented by the io.jmix.uiexport.action.ExcelExportAction
class and should be defined in XML using type="excelExport"
action’s attribute for a list component. You can configure common action parameters using XML attributes of the action
element. See Declarative Actions for details.
For example:
<actions>
<action id="excelExport" type="excelExport"/>
</actions>
Alternatively, you can inject the action into the screen controller and configure it using setters:
@Named("customersTable.excel")
protected ExcelExportAction customersTableExcel;
@Subscribe
protected void onInit(InitEvent event) {
customersTableExcel.setCaption("Export to Excel");
}
You can override localized data format strings. The default set of format strings, defined for the excel export, is the following:
excelExporter.true=Yes
excelExporter.false=No
excelExporter.empty=[Empty]
excelExporter.bytes=<bytes>
excelExporter.timeFormat=h:mm
excelExporter.dateFormat=m/d/yy
excelExporter.dateTimeFormat=m/d/yy h:mm
excelExporter.integerFormat=#,##0
excelExporter.doubleFormat=#,##0.00##############
The XLS format implies the limitation for 65536 table rows. If an exported table contains more than 65536 rows, its content will be cut by the last row, and you will see the corresponding warning message. |
JsonExportAction
JsonExportAction
is an action extending ExportAction and designed to export the table content in JSON format.
The action is implemented by the io.jmix.uiexport.action.JsonExportAction
class and should be defined in XML using type="jsonExport"
action’s attribute for a list component. You can configure common action parameters using XML attributes of the action
element. See Declarative Actions for details.
For example:
<actions>
<action id="jsonExport" type="jsonExport"/>
</actions>
Alternatively, you can inject the action into the screen controller and configure it using setters:
@Named("customersTable.json")
protected JsonExportAction customersTableJson;
@Subscribe
protected void onInit(InitEvent event) {
customersTableJson.setCaption("Export to JSON");
}